-
Notifications
You must be signed in to change notification settings - Fork 98
New issue
Have a question about this project? Sign up for a free GitHub account to open an issue and contact its maintainers and the community.
By clicking “Sign up for GitHub”, you agree to our terms of service and privacy statement. We’ll occasionally send you account related emails.
Already on GitHub? Sign in to your account
Generic Dialogs API spec #94
Comments
Looks really good and it allows to make our applications even better. |
I really like this, as it adds more customisation options. alot like the material.avalonia custom dialogs |
FYI, similar ideas were discussed in WinUI here: microsoft/microsoft-ui-xaml#2313. You might find some inspiration/good-ideas there as well. |
Looks like Avalonia has a discussion as well: AvaloniaUI/Avalonia#670 (comment) |
Can be closed as #104 has merged |
I've had a couple requests for enhancements to the existing
ContentDialog
functionality. As a result, I'm drafting up an API spec for generic dialogs. AsContentDialog
is a WinUI item (reverse engineered instead of ported, but still) I don't want to modify the API in a way that makes it different to the upstream version other than bug fixes or minor feature additions.The biggest issue from the comments is that the lack of button options in
ContentDialog
make it somewhat limiting, something the old vista-styleTaskDialog
solves (to an extent). This proposal, therefore, seeks a new control and API spec. Note that this is just an API spec to layout the general thought and get feedback of desired features - and is not yet a firm proposal or commitment.EDIT: Final spec laid out here - PR created
Final design:
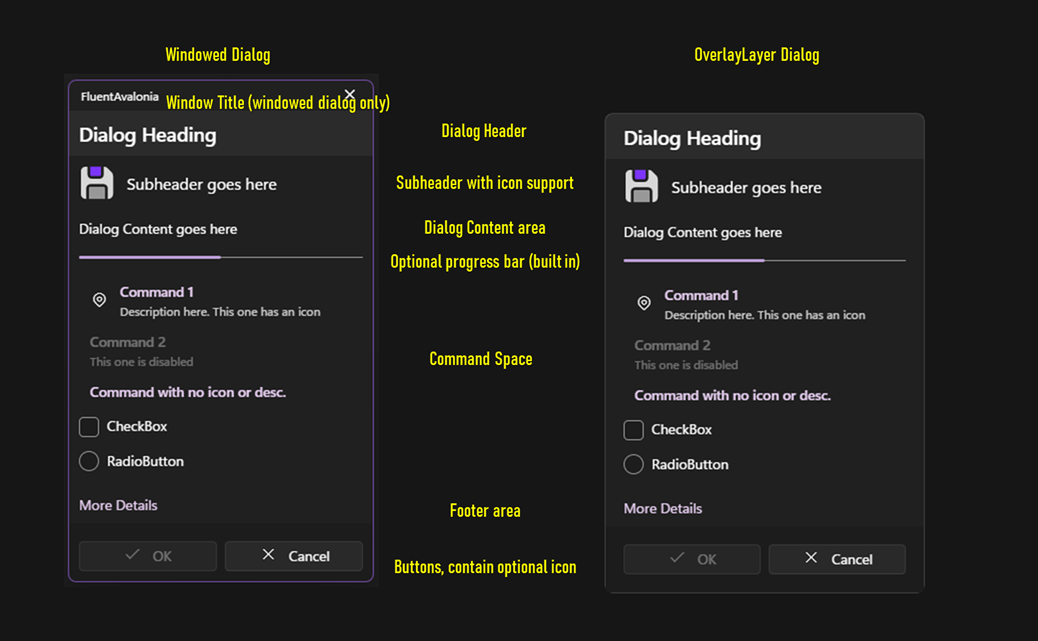
The text was updated successfully, but these errors were encountered: