From 5d98874e8fbfde9188090c1b93eeb62cc141f397 Mon Sep 17 00:00:00 2001
From: Lee Robinson
Date: Mon, 18 Jan 2021 17:37:32 -0600
Subject: [PATCH 1/5] Add guide "Migrating from Create React App" (#21212)
---
docs/manifest.json | 8 +
docs/migrating/from-create-react-app.md | 238 ++++++++++++++++++++++++
2 files changed, 246 insertions(+)
create mode 100644 docs/migrating/from-create-react-app.md
diff --git a/docs/manifest.json b/docs/manifest.json
index 6f248588ba11f..2744470517ebc 100644
--- a/docs/manifest.json
+++ b/docs/manifest.json
@@ -213,6 +213,14 @@
{
"title": "Migrating from Gatsby",
"path": "/docs/migrating/from-gatsby.md"
+ },
+ {
+ "title": "Migrating from Create React App",
+ "path": "/docs/migrating/from-create-react-app.md"
+ },
+ {
+ "title": "Migrating from React Router",
+ "path": "/docs/migrating/from-react-router.md"
}
]
},
diff --git a/docs/migrating/from-create-react-app.md b/docs/migrating/from-create-react-app.md
new file mode 100644
index 0000000000000..b8eba77af8777
--- /dev/null
+++ b/docs/migrating/from-create-react-app.md
@@ -0,0 +1,238 @@
+---
+description: Learn how to transition an existing Create React App project to Next.js.
+---
+
+# Migrating from Create React App
+
+This guide will help you understand how to transition from an existing non-ejected Create React App project to Next.js. Migrating to Next.js will allow you to:
+
+- Choose which [data fetching](/docs/basic-features/data-fetching.md) strategy you want on a per-page basis.
+- Use [Incremental Static Regeneration](/docs/basic-features/data-fetching.md#incremental-static-regeneration) to update _existing_ pages by re-rendering them in the background as traffic comes in.
+- Use [API Routes](/docs/api-routes/introduction.md).
+
+And more! Let’s walk through a series of steps to complete the migration.
+
+## Updating `package.json` and dependencies
+
+The first step towards migrating to Next.js is to update `package.json` and dependencies. You should:
+
+- Remove `react-scripts` (but keep `react` and `react-dom`). If you're using React Router, you can also remove `react-router-dom`.
+- Install `next`.
+- Add Next.js related commands to `scripts`. One is `next dev`, which runs a development server at `localhost:3000`. You should also add `next build` and `next start` for creating and starting a production build.
+
+Here's an example `package.json`:
+
+```json
+{
+ "scripts": {
+ "dev": "next dev",
+ "build": "next build",
+ "start": "next start"
+ },
+ "dependencies": {
+ "next": "latest",
+ "react": "latest",
+ "react-dom": "latest"
+ }
+}
+```
+
+## Static Assets and Compiled Output
+
+Create React App uses the `public` directory for the [entry HTML file](https://create-react-app.dev/docs/using-the-public-folder), whereas Next.js uses it for static assets. It's possible to add static assets here, but Create React App recommends importing them directly from JavaScript files.
+
+- Move any images, fonts, or other static assets to `public`.
+- Convert `index.html` (the entry point of your application) to Next.js. Any `` code should be moved to a [custom `_document.js`](/docs/advanced-features/custom-document.md). Any shared layout between all pages should be moved to a [custom `_app.js`](/docs/advanced-features/custom-app.md).
+- See [Styling](#styling) for CSS/Sass files.
+- Add `.next` to `.gitignore`.
+
+## Creating Routes & Linking
+
+With Create React App, you're likely using React Router. Instead of using a third-party library, Next.js includes it's own [file-system based routing](/docs/routing/introduction.md).
+
+- Convert all `Route` components to new files in the `pages` directory.
+- For routes that require dynamic content (e.g. `/blog/:slug`), you can use [Dynamic Routes](/docs/routing/dynamic-routes.md) with Next.js (e.g. `pages/blog/[slug].js`). The value of `slug` is accessible through a [query parameter](/docs/routing/dynamic-routes.md). For example, the route `/blog/first-post` would forward the query object `{ 'slug': 'first-post' }` to `pages/blog/[slug].js` ([learn more here](/docs/basic-features/data-fetching.md#getstaticpaths-static-generation)).
+
+For more information, see [Migrating from React Router](/docs/migrating/from-react-router.md).
+
+## Styling
+
+Next.js has built-in support for [CSS](/docs/basic-features/built-in-css-support.md), [Sass](/docs/basic-features/built-in-css-support.md#sass-support) and [CSS-in-JS](/docs/basic-features/built-in-css-support.md#css-in-js).
+
+With Create React App, you can import `.css` files directly inside React components. Next.js allows you to do the same, but requires these files to be [CSS Modules](/docs/basic-features/built-in-css-support.md). For global styles, you'll need a [custom `_app.js`](/docs/advanced-features/custom-app.md) to add a [global stylesheet](docs/basic-features/built-in-css-support.md#adding-a-global-stylesheet).
+
+## Safely Accessing Web APIs
+
+With client-side rendered applications (like Create React App), you can access `window`, `localStorage`, `navigator`, and other [Web APIs](https://developer.mozilla.org/en-US/docs/Web/API) out of the box.
+
+Since Next.js uses [pre-rendering](/docs/basic-features/pages.md#pre-rendering), you'll need to safely access those Web APIs only when you're on the client-side. For example, the following code snippet will allow access to `window` only on the client-side.
+
+```jsx
+if (typeof window !== 'undefined') {
+ // You now have access to `window`
+}
+```
+
+A recommended way of accessing Web APIs safely is by using the [`useEffect`](https://reactjs.org/docs/hooks-effect.html) hook, which only executes client-side:
+
+```jsx
+import { useEffect } from 'react'
+
+useEffect(() => {
+ // You now have access to `window`
+}, [])
+```
+## Image Component and Image Optimization
+
+Since version **10.0.0**, Next.js has a built-in [Image Component and Automatic Image Optimization](/docs/basic-features/image-optimization.md).
+
+The Next.js Image Component, [`next/image`](/docs/api-reference/next/image.md), is an extension of the HTML `` element, evolved for the modern web.
+
+The Automatic Image Optimization allows for resizing, optimizing, and serving images in modern formats like [WebP](https://developer.mozilla.org/en-US/docs/Web/Media/Formats/Image_types) when the browser supports it. This avoids shipping large images to devices with a smaller viewport. It also allows Next.js to automatically adopt future image formats and serve them to browsers that support those formats.
+
+Instead of optimizing images at build time, Next.js optimizes images on-demand, as users request them. Your build times aren't increased, whether shipping 10 images or 10 million images.
+
+```jsx
+import Image from 'next/image'
+
+export default function Home() {
+ return (
+ <>
+
My Homepage
+
+
Welcome to my homepage!
+ >
+ )
+}
+```
+
+## Environment Variables
+
+Next.js has support for `.env` [Environment Variables](/docs/basic-features/environment-variables.md) similar to Create React App. The main different is the prefix used to expose environment variables on the client-side.
+
+- Change all environment variables with the `REACT_APP_` prefix to `NEXT_PUBLIC_`.
+- Server-side environment variables will be available at build-time and in [API Routes](/docs/api-routes/introduction.md).
+
+## Search Engine Optimization
+
+Most Create React App examples use `react-helmet` to assist with adding `meta` tags for proper SEO. With Next.js, we use [`next/head`](/docs/api-reference/next/head.md) to add `meta` tags to your `` element. For example, here's an SEO component with Create React App:
+
+```jsx
+// src/components/seo.js
+
+import { Helmet } from 'react-helmet'
+
+export default function SEO({ description, title, siteTitle }) {
+ return (
+
+ )
+}
+```
+
+And here's the same example using Next.js.
+
+```jsx
+// src/components/seo.js
+
+import Head from 'next/head'
+
+export default function SEO({ description, title, siteTitle }) {
+ return (
+
+ {`${title} | ${siteTitle}`}
+
+
+
+
+
+
+
+
+
+
+ )
+}
+```
+
+## Single-Page App (SPA)
+
+If you want to move your existing Create React App to Next.js and keep a Single-Page App, you can move your old application entry point to an [Optional Catch-All Route](/docs/routing/dynamic-routes.md#optional-catch-all-routes) named `pages/[[…app]].js`.
+
+```jsx
+// pages/[[...app]].js
+
+import { useState } from 'react'
+import CreateReactAppEntryPoint from '../components/app'
+
+function App() {
+ const [isMounted, setIsMounted] = useState(false)
+
+ useEffect(() => {
+ setIsMounted(true)
+ }, [])
+
+ if (!isMounted) {
+ return null
+ }
+
+ return
+}
+
+export default App
+```
+
+## Ejected Create React App
+
+If you've ejected Create React App, here are some things to consider:
+
+- If you have custom file loaders set up for CSS, Sass, or other assets, this is all built-in with Next.js.
+- If you've manually added [new JavaScript features](/docs/basic-features/supported-browsers-features.md#javascript-language-features) (e.g. Optional Chaining) or [Polyfills](/docs/basic-features/supported-browsers-features.md#polyfills), check to see what's included by default with Next.js.
+- If you have a custom code splitting setup, you can remove that. Next.js has automatic code splitting on a [per-page basis](/docs/basic-features/pages.md).
+- You can [customize your PostCSS setup](/docs/advanced-features/customizing-postcss-config.md#default-behavior) with Next.js without ejecting from the framework.
+- You should reference the default [Babel config](/docs/advanced-features/customizing-babel-config.md) and [Webpack config](/docs/api-reference/next.config.js/custom-webpack-config.md) of Next.js to see what's included by default.
+
+## Learn More
+
+You can learn more about Next.js by completing our [starter tutorial](https://nextjs.org/learn/basics/getting-started). If you have questions or if this guide didn't work for you, feel free to reach out to our community on [GitHub Discussions](https://github.com/vercel/next.js/discussions).
From cfae5c6c32ff642b0b71dafe48182783699a66f1 Mon Sep 17 00:00:00 2001
From: Joe Haddad
Date: Tue, 19 Jan 2021 00:26:53 -0500
Subject: [PATCH 2/5] fix: lint
---
docs/migrating/from-create-react-app.md | 1 +
1 file changed, 1 insertion(+)
diff --git a/docs/migrating/from-create-react-app.md b/docs/migrating/from-create-react-app.md
index b8eba77af8777..03c977693b1de 100644
--- a/docs/migrating/from-create-react-app.md
+++ b/docs/migrating/from-create-react-app.md
@@ -82,6 +82,7 @@ useEffect(() => {
// You now have access to `window`
}, [])
```
+
## Image Component and Image Optimization
Since version **10.0.0**, Next.js has a built-in [Image Component and Automatic Image Optimization](/docs/basic-features/image-optimization.md).
From e28fd50441cfaa22554db2b8e5bb7d03061e67d4 Mon Sep 17 00:00:00 2001
From: Luis Alvarez D
Date: Tue, 19 Jan 2021 02:28:54 -0500
Subject: [PATCH 3/5] Include utm_source on example links to vercel.com
(#21305)
---
examples/active-class-name/README.md | 2 +-
examples/amp-first/README.md | 2 +-
examples/amp-story/README.md | 2 +-
examples/amp/README.md | 2 +-
examples/analyze-bundles/README.md | 2 +-
examples/api-routes-apollo-server-and-client-auth/README.md | 2 +-
examples/api-routes-apollo-server/README.md | 2 +-
examples/api-routes-cors/README.md | 2 +-
examples/api-routes-graphql/README.md | 2 +-
examples/api-routes-middleware/README.md | 2 +-
examples/api-routes-rate-limit/README.md | 2 +-
examples/api-routes-rest/README.md | 2 +-
examples/api-routes/README.md | 2 +-
examples/basic-css/README.md | 2 +-
examples/basic-export/README.md | 2 +-
examples/catch-all-routes/README.md | 2 +-
examples/data-fetch/README.md | 2 +-
examples/dynamic-routing/README.md | 2 +-
examples/environment-variables/readme.md | 2 +-
examples/fast-refresh-demo/README.md | 2 +-
examples/head-elements/README.md | 2 +-
examples/hello-world/README.md | 2 +-
examples/i18n-routing/README.md | 2 +-
examples/image-component/README.md | 2 +-
examples/layout-component/README.md | 2 +-
examples/nested-components/README.md | 2 +-
examples/progressive-render/README.md | 2 +-
examples/progressive-web-app/README.md | 2 +-
examples/redirects/README.md | 2 +-
examples/rewrites/README.md | 2 +-
examples/svg-components/README.md | 2 +-
examples/using-router/README.md | 2 +-
examples/with-absolute-imports/README.md | 2 +-
examples/with-ant-design-less/README.md | 2 +-
examples/with-ant-design-mobile/README.md | 2 +-
examples/with-ant-design-pro-layout-less/README.md | 2 +-
examples/with-ant-design/README.md | 2 +-
examples/with-aphrodite/README.md | 2 +-
examples/with-apollo-and-redux/README.md | 2 +-
examples/with-apollo/README.md | 2 +-
examples/with-app-layout/README.md | 2 +-
examples/with-astroturf/README.md | 2 +-
examples/with-babel-macros/README.md | 2 +-
examples/with-carbon-components/README.md | 2 +-
examples/with-cerebral/README.md | 2 +-
examples/with-chakra-ui-typescript/README.md | 2 +-
examples/with-chakra-ui/README.md | 2 +-
examples/with-context-api/README.md | 2 +-
examples/with-cssed/README.md | 2 +-
examples/with-custom-babel-config/README.md | 2 +-
examples/with-cxs/README.md | 2 +-
examples/with-draft-js/README.md | 2 +-
examples/with-dynamic-app-layout/README.md | 2 +-
examples/with-dynamic-import/README.md | 2 +-
examples/with-emotion/README.md | 2 +-
examples/with-env-from-next-config-js/README.md | 2 +-
examples/with-expo-typescript/README.md | 2 +-
examples/with-expo/README.md | 2 +-
examples/with-facebook-pixel/README.md | 2 +-
examples/with-fela/README.md | 2 +-
examples/with-filbert/README.md | 2 +-
examples/with-firebase/README.md | 2 +-
examples/with-flow/README.md | 2 +-
examples/with-framer-motion/README.md | 2 +-
examples/with-glamor/README.md | 2 +-
examples/with-google-analytics-amp/README.md | 2 +-
examples/with-google-analytics/README.md | 2 +-
examples/with-google-tag-manager/README.md | 2 +-
examples/with-graphql-faunadb/README.md | 2 +-
examples/with-graphql-hooks/README.md | 2 +-
examples/with-graphql-react/README.md | 2 +-
examples/with-grommet/README.md | 2 +-
examples/with-gsap/README.md | 2 +-
examples/with-i18n-rosetta/README.md | 2 +-
examples/with-ionic-typescript/README.md | 2 +-
examples/with-iron-session/README.md | 2 +-
examples/with-kea/README.md | 2 +-
examples/with-linaria/README.md | 2 +-
examples/with-lingui/README.md | 2 +-
examples/with-loading/README.md | 2 +-
examples/with-mdbreact/README.md | 2 +-
examples/with-mdx-remote/README.md | 2 +-
examples/with-mdx/README.md | 2 +-
examples/with-mobx-react-lite/README.md | 2 +-
examples/with-mobx-state-tree-typescript/README.md | 2 +-
examples/with-mobx-state-tree/README.md | 2 +-
examples/with-mobx/README.md | 2 +-
examples/with-monaco-editor/README.md | 2 +-
examples/with-mongodb-mongoose/README.md | 2 +-
examples/with-mqtt-js/README.md | 2 +-
examples/with-msw/README.md | 2 +-
examples/with-next-css/README.md | 2 +-
examples/with-next-less/README.md | 2 +-
examples/with-next-page-transitions/README.md | 2 +-
examples/with-next-sass/README.md | 2 +-
examples/with-next-seo/README.md | 2 +-
examples/with-next-sitemap/README.md | 2 +-
examples/with-next-translate/README.md | 2 +-
examples/with-orbit-components/README.md | 2 +-
examples/with-overmind/README.md | 2 +-
examples/with-passport-and-next-connect/README.md | 2 +-
examples/with-passport/README.md | 2 +-
examples/with-paste-typescript/README.md | 2 +-
examples/with-patternfly/README.md | 2 +-
examples/with-polyfills/README.md | 2 +-
examples/with-portals-ssr/README.md | 2 +-
examples/with-portals/README.md | 2 +-
examples/with-prefetching/README.md | 2 +-
examples/with-quill-js/README.md | 2 +-
examples/with-rbx-bulma-pro/README.md | 2 +-
examples/with-react-bootstrap/README.md | 2 +-
examples/with-react-ga/README.md | 2 +-
examples/with-react-helmet/README.md | 2 +-
examples/with-react-jss/README.md | 2 +-
examples/with-react-multi-carousel/README.md | 2 +-
examples/with-react-native-web/README.md | 2 +-
examples/with-react-relay-network-modern/README.md | 2 +-
examples/with-react-toolbox/README.md | 2 +-
examples/with-react-with-styles/README.md | 2 +-
examples/with-reactstrap/README.md | 2 +-
examples/with-realm-web/README.md | 2 +-
examples/with-reason-relay/README.md | 2 +-
examples/with-reasonml-todo/README.md | 2 +-
examples/with-reasonml/README.md | 2 +-
examples/with-rebass/README.md | 2 +-
examples/with-recoil/README.md | 2 +-
examples/with-redux-code-splitting/README.md | 2 +-
examples/with-redux-observable/README.md | 2 +-
examples/with-redux-persist/README.md | 2 +-
examples/with-redux-saga/README.md | 2 +-
examples/with-redux-thunk/README.md | 2 +-
examples/with-redux-toolkit/README.md | 2 +-
examples/with-redux-wrapper/README.md | 2 +-
examples/with-redux/README.md | 2 +-
examples/with-reflux/README.md | 2 +-
examples/with-relay-modern/README.md | 2 +-
examples/with-rematch/README.md | 2 +-
examples/with-route-as-modal/README.md | 2 +-
examples/with-segment-analytics/README.md | 2 +-
examples/with-semantic-ui/README.md | 2 +-
examples/with-shallow-routing/README.md | 2 +-
examples/with-sitemap/README.md | 2 +-
examples/with-slate/README.md | 2 +-
examples/with-stencil/README.md | 2 +-
examples/with-stitches/README.md | 2 +-
examples/with-storybook-styled-jsx-scss/README.md | 2 +-
examples/with-storybook/README.md | 2 +-
examples/with-strict-csp/README.md | 2 +-
examples/with-style-sheet/README.md | 2 +-
examples/with-styled-components-rtl/README.md | 2 +-
examples/with-styled-components/README.md | 2 +-
examples/with-styled-jsx-plugins/README.md | 2 +-
examples/with-styled-jsx-scss/README.md | 2 +-
examples/with-styled-jsx/README.md | 2 +-
examples/with-styletron/README.md | 2 +-
examples/with-tailwindcss-emotion/README.md | 2 +-
examples/with-tailwindcss/README.md | 2 +-
examples/with-tesfy/README.md | 2 +-
examples/with-three-js/README.md | 2 +-
examples/with-typescript-eslint-jest/README.md | 2 +-
examples/with-typescript-graphql/README.md | 2 +-
examples/with-typescript-styled-components/README.md | 2 +-
examples/with-typescript/README.md | 2 +-
examples/with-typestyle/README.md | 2 +-
examples/with-unstated/README.md | 2 +-
examples/with-videojs/README.md | 2 +-
examples/with-why-did-you-render/README.md | 2 +-
examples/with-xstate/README.md | 2 +-
examples/with-yarn-workspaces/README.md | 2 +-
examples/with-zeit-fetch/README.md | 2 +-
examples/with-zustand/README.md | 2 +-
171 files changed, 171 insertions(+), 171 deletions(-)
diff --git a/examples/active-class-name/README.md b/examples/active-class-name/README.md
index aa548694cb9a3..03d46cea4d39e 100644
--- a/examples/active-class-name/README.md
+++ b/examples/active-class-name/README.md
@@ -4,7 +4,7 @@ ReactRouter has a convenience property on the `Link` element to allow an author
## Deploy your own
-Deploy the example using [Vercel](https://vercel.com):
+Deploy the example using [Vercel](https://vercel.com?utm_source=github&utm_medium=readme&utm_campaign=next-example):
[](https://vercel.com/new/git/external?repository-url=https://github.com/vercel/next.js/tree/canary/examples/active-class-name&project-name=active-class-name&repository-name=active-class-name)
diff --git a/examples/amp-first/README.md b/examples/amp-first/README.md
index 9dc2a3e81084e..1213e4471715e 100644
--- a/examples/amp-first/README.md
+++ b/examples/amp-first/README.md
@@ -9,7 +9,7 @@ This example sets up the boilerplate for an AMP First Site. You can see a live v
## Deploy your own
-Deploy the example using [Vercel](https://vercel.com):
+Deploy the example using [Vercel](https://vercel.com?utm_source=github&utm_medium=readme&utm_campaign=next-example):
[](https://vercel.com/new/git/external?repository-url=https://github.com/vercel/next.js/tree/canary/examples/amp-first&project-name=amp-first&repository-name=amp-first)
diff --git a/examples/amp-story/README.md b/examples/amp-story/README.md
index 4398d14bea141..79cb3ae066180 100644
--- a/examples/amp-story/README.md
+++ b/examples/amp-story/README.md
@@ -4,7 +4,7 @@ This example shows how to create an AMP page with `amp-story` using Next.js and
## Deploy your own
-Deploy the example using [Vercel](https://vercel.com):
+Deploy the example using [Vercel](https://vercel.com?utm_source=github&utm_medium=readme&utm_campaign=next-example):
[](https://vercel.com/new/git/external?repository-url=https://github.com/vercel/next.js/tree/canary/examples/amp-story&project-name=amp-story&repository-name=amp-story)
diff --git a/examples/amp/README.md b/examples/amp/README.md
index 34c5884ae57ea..33aa31b3684e5 100644
--- a/examples/amp/README.md
+++ b/examples/amp/README.md
@@ -4,7 +4,7 @@ This example shows how to create AMP pages using Next.js and the AMP feature. It
## Deploy your own
-Deploy the example using [Vercel](https://vercel.com):
+Deploy the example using [Vercel](https://vercel.com?utm_source=github&utm_medium=readme&utm_campaign=next-example):
[](https://vercel.com/new/git/external?repository-url=https://github.com/vercel/next.js/tree/canary/examples/amp&project-name=amp&repository-name=amp)
diff --git a/examples/analyze-bundles/README.md b/examples/analyze-bundles/README.md
index f635f724134cf..abea34df8353d 100644
--- a/examples/analyze-bundles/README.md
+++ b/examples/analyze-bundles/README.md
@@ -4,7 +4,7 @@ This example shows how to analyze the output bundles using [@next/bundle-analyze
## Deploy your own
-Deploy the example using [Vercel](https://vercel.com):
+Deploy the example using [Vercel](https://vercel.com?utm_source=github&utm_medium=readme&utm_campaign=next-example):
[](https://vercel.com/new/git/external?repository-url=https://github.com/vercel/next.js/tree/canary/examples/analyze-bundles&project-name=analyze-bundles&repository-name=analyze-bundles)
diff --git a/examples/api-routes-apollo-server-and-client-auth/README.md b/examples/api-routes-apollo-server-and-client-auth/README.md
index 0a80c1a0144ec..68ca3be46917e 100644
--- a/examples/api-routes-apollo-server-and-client-auth/README.md
+++ b/examples/api-routes-apollo-server-and-client-auth/README.md
@@ -6,7 +6,7 @@ In this simple example, we integrate Apollo seamlessly with [Next.js data fetchi
## Deploy your own
-Deploy the example using [Vercel](https://vercel.com):
+Deploy the example using [Vercel](https://vercel.com?utm_source=github&utm_medium=readme&utm_campaign=next-example):
[](https://vercel.com/new/git/external?repository-url=https://github.com/vercel/next.js/tree/canary/examples/api-routes-apollo-server-and-client-auth&project-name=api-routes-apollo-server-and-client-auth&repository-name=api-routes-apollo-server-and-client-auth)
diff --git a/examples/api-routes-apollo-server/README.md b/examples/api-routes-apollo-server/README.md
index dc9ebb1897a59..320060be87784 100644
--- a/examples/api-routes-apollo-server/README.md
+++ b/examples/api-routes-apollo-server/README.md
@@ -4,7 +4,7 @@ Next.js ships with two forms of pre-rendering: [Static Generation](https://nextj
## Deploy your own
-Deploy the example using [Vercel](https://vercel.com):
+Deploy the example using [Vercel](https://vercel.com?utm_source=github&utm_medium=readme&utm_campaign=next-example):
[](https://vercel.com/new/git/external?repository-url=https://github.com/vercel/next.js/tree/canary/examples/api-routes-apollo-server&project-name=api-routes-apollo-server&repository-name=api-routes-apollo-server)
diff --git a/examples/api-routes-cors/README.md b/examples/api-routes-cors/README.md
index 4a8d29da41526..5fdee8ab744b8 100644
--- a/examples/api-routes-cors/README.md
+++ b/examples/api-routes-cors/README.md
@@ -6,7 +6,7 @@ This example shows how to create an `API` endpoint with [CORS](https://developer
## Deploy your own
-Deploy the example using [Vercel](https://vercel.com):
+Deploy the example using [Vercel](https://vercel.com?utm_source=github&utm_medium=readme&utm_campaign=next-example):
[](https://vercel.com/new/git/external?repository-url=https://github.com/vercel/next.js/tree/canary/examples/api-routes-cors&project-name=api-routes-cors&repository-name=api-routes-cors)
diff --git a/examples/api-routes-graphql/README.md b/examples/api-routes-graphql/README.md
index 64db55ce74a42..1a31922c94a90 100644
--- a/examples/api-routes-graphql/README.md
+++ b/examples/api-routes-graphql/README.md
@@ -4,7 +4,7 @@ Next.js ships with [API routes](https://github.com/vercel/next.js#api-routes), w
## Deploy your own
-Deploy the example using [Vercel](https://vercel.com):
+Deploy the example using [Vercel](https://vercel.com?utm_source=github&utm_medium=readme&utm_campaign=next-example):
[](https://vercel.com/new/git/external?repository-url=https://github.com/vercel/next.js/tree/canary/examples/api-routes-graphql&project-name=api-routes-graphql&repository-name=api-routes-graphql)
diff --git a/examples/api-routes-middleware/README.md b/examples/api-routes-middleware/README.md
index 831f06f552635..7ed15b6ff90a5 100644
--- a/examples/api-routes-middleware/README.md
+++ b/examples/api-routes-middleware/README.md
@@ -4,7 +4,7 @@ Next.js ships with [API routes](https://github.com/vercel/next.js#api-routes), w
## Deploy your own
-Deploy the example using [Vercel](https://vercel.com):
+Deploy the example using [Vercel](https://vercel.com?utm_source=github&utm_medium=readme&utm_campaign=next-example):
[](https://vercel.com/new/git/external?repository-url=https://github.com/vercel/next.js/tree/canary/examples/api-routes-middleware&project-name=api-routes-middleware&repository-name=api-routes-middleware)
diff --git a/examples/api-routes-rate-limit/README.md b/examples/api-routes-rate-limit/README.md
index 1d8bc8aabc9b3..e3397b2a9fc4e 100644
--- a/examples/api-routes-rate-limit/README.md
+++ b/examples/api-routes-rate-limit/README.md
@@ -18,7 +18,7 @@ X-RateLimit-Remaining: 0
## Deploy your own
-Deploy the example using [Vercel](https://vercel.com):
+Deploy the example using [Vercel](https://vercel.com?utm_source=github&utm_medium=readme&utm_campaign=next-example):
[](https://vercel.com/new/git/external?repository-url=https://github.com/vercel/next.js/tree/canary/examples/api-routes-rate-limit&project-name=api-routes-rate-limit&repository-name=api-routes-rate-limit)
diff --git a/examples/api-routes-rest/README.md b/examples/api-routes-rest/README.md
index bcf634495e8dc..5183ca2166520 100644
--- a/examples/api-routes-rest/README.md
+++ b/examples/api-routes-rest/README.md
@@ -4,7 +4,7 @@ Next.js ships with [API routes](https://github.com/vercel/next.js#api-routes), w
## Deploy your own
-Deploy the example using [Vercel](https://vercel.com):
+Deploy the example using [Vercel](https://vercel.com?utm_source=github&utm_medium=readme&utm_campaign=next-example):
[](https://vercel.com/new/git/external?repository-url=https://github.com/vercel/next.js/tree/canary/examples/api-routes-rest&project-name=api-routes-rest&repository-name=api-routes-rest)
diff --git a/examples/api-routes/README.md b/examples/api-routes/README.md
index 19951ef3d7352..334bf15feedb0 100644
--- a/examples/api-routes/README.md
+++ b/examples/api-routes/README.md
@@ -4,7 +4,7 @@ Next.js ships with [API routes](https://nextjs.org/docs/api-routes/introduction)
## Deploy your own
-Deploy the example using [Vercel](https://vercel.com):
+Deploy the example using [Vercel](https://vercel.com?utm_source=github&utm_medium=readme&utm_campaign=next-example):
[](https://vercel.com/new/git/external?repository-url=https://github.com/vercel/next.js/tree/canary/examples/api-routes&project-name=api-routes&repository-name=api-routes)
diff --git a/examples/basic-css/README.md b/examples/basic-css/README.md
index 5b6134bdde271..a7e56f8b5f0dc 100644
--- a/examples/basic-css/README.md
+++ b/examples/basic-css/README.md
@@ -4,7 +4,7 @@ Next.js has built-in support for [CSS Modules](https://nextjs.org/docs/basic-fea
## Deploy your own
-Deploy the example using [Vercel](https://vercel.com):
+Deploy the example using [Vercel](https://vercel.com?utm_source=github&utm_medium=readme&utm_campaign=next-example):
[](https://vercel.com/new/git/external?repository-url=https://github.com/vercel/next.js/tree/canary/examples/basic-css&project-name=basic-css&repository-name=basic-css)
diff --git a/examples/basic-export/README.md b/examples/basic-export/README.md
index 4fe9c89faa666..06f34a95483e2 100644
--- a/examples/basic-export/README.md
+++ b/examples/basic-export/README.md
@@ -4,7 +4,7 @@ This example shows the most basic usage of `next export`. Without `exportPathMap
## Deploy your own
-Deploy the example using [Vercel](https://vercel.com):
+Deploy the example using [Vercel](https://vercel.com?utm_source=github&utm_medium=readme&utm_campaign=next-example):
[](https://vercel.com/new/git/external?repository-url=https://github.com/vercel/next.js/tree/canary/examples/basic-export&project-name=basic-export&repository-name=basic-export)
diff --git a/examples/catch-all-routes/README.md b/examples/catch-all-routes/README.md
index 61ff50f23b247..36e1968d58861 100644
--- a/examples/catch-all-routes/README.md
+++ b/examples/catch-all-routes/README.md
@@ -13,7 +13,7 @@ You can use `next/link` as displayed in this example to route to these pages cli
## Deploy your own
-Deploy the example using [Vercel](https://vercel.com):
+Deploy the example using [Vercel](https://vercel.com?utm_source=github&utm_medium=readme&utm_campaign=next-example):
[](https://vercel.com/new/git/external?repository-url=https://github.com/vercel/next.js/tree/canary/examples/catch-all-routes&project-name=catch-all-routes&repository-name=catch-all-routes)
diff --git a/examples/data-fetch/README.md b/examples/data-fetch/README.md
index c9f9b67c7539a..e47745a3fe955 100644
--- a/examples/data-fetch/README.md
+++ b/examples/data-fetch/README.md
@@ -7,7 +7,7 @@ By using `getStaticProps` Next.js will fetch data at build time from a page, and
## Deploy your own
-Deploy the example using [Vercel](https://vercel.com):
+Deploy the example using [Vercel](https://vercel.com?utm_source=github&utm_medium=readme&utm_campaign=next-example):
[](https://vercel.com/new/git/external?repository-url=https://github.com/vercel/next.js/tree/canary/examples/data-fetch&project-name=data-fetch&repository-name=data-fetch)
diff --git a/examples/dynamic-routing/README.md b/examples/dynamic-routing/README.md
index d204c8964b6f5..aac91caf04318 100644
--- a/examples/dynamic-routing/README.md
+++ b/examples/dynamic-routing/README.md
@@ -12,7 +12,7 @@ You can use `next/link` as displayed in this example to route to these pages cli
## Deploy your own
-Deploy the example using [Vercel](https://vercel.com):
+Deploy the example using [Vercel](https://vercel.com?utm_source=github&utm_medium=readme&utm_campaign=next-example):
[](https://vercel.com/new/git/external?repository-url=https://github.com/vercel/next.js/tree/canary/examples/dynamic-routing&project-name=dynamic-routing&repository-name=dynamic-routing)
diff --git a/examples/environment-variables/readme.md b/examples/environment-variables/readme.md
index 62f842bc43934..6a3b50bce7eed 100644
--- a/examples/environment-variables/readme.md
+++ b/examples/environment-variables/readme.md
@@ -6,7 +6,7 @@ The index page ([pages/index.js](pages/index.js)) will show you how to [access e
## Deploy your own
-Deploy the example using [Vercel](https://vercel.com):
+Deploy the example using [Vercel](https://vercel.com?utm_source=github&utm_medium=readme&utm_campaign=next-example):
[](https://vercel.com/new/git/external?repository-url=https://github.com/vercel/next.js/tree/canary/examples/environment-variables&project-name=environment-variables&repository-name=environment-variables)
diff --git a/examples/fast-refresh-demo/README.md b/examples/fast-refresh-demo/README.md
index fa2cb417f2cd0..268b7f86ca285 100644
--- a/examples/fast-refresh-demo/README.md
+++ b/examples/fast-refresh-demo/README.md
@@ -6,7 +6,7 @@ This demos shows how the state of an auto incrementing value and a classic count
## Deploy your own
-Deploy the example using [Vercel](https://vercel.com):
+Deploy the example using [Vercel](https://vercel.com?utm_source=github&utm_medium=readme&utm_campaign=next-example):
[](https://vercel.com/new/git/external?repository-url=https://github.com/vercel/next.js/tree/canary/examples/fast-refresh-demo&project-name=fast-refresh-demo&repository-name=fast-refresh-demo)
diff --git a/examples/head-elements/README.md b/examples/head-elements/README.md
index b3c0c6d653ff5..61f9b53b76ee2 100644
--- a/examples/head-elements/README.md
+++ b/examples/head-elements/README.md
@@ -6,7 +6,7 @@ This example shows in `pages/index.js` how to add a title and a couple of meta t
## Deploy your own
-Deploy the example using [Vercel](https://vercel.com):
+Deploy the example using [Vercel](https://vercel.com?utm_source=github&utm_medium=readme&utm_campaign=next-example):
[](https://vercel.com/new/git/external?repository-url=https://github.com/vercel/next.js/tree/canary/examples/head-elements&project-name=head-elements&repository-name=head-elements)
diff --git a/examples/hello-world/README.md b/examples/hello-world/README.md
index 3b37739dfdf73..9008bdc23e71b 100644
--- a/examples/hello-world/README.md
+++ b/examples/hello-world/README.md
@@ -4,7 +4,7 @@ This example shows the most basic idea behind Next. We have 2 pages: `pages/inde
## Deploy your own
-Deploy the example using [Vercel](https://vercel.com):
+Deploy the example using [Vercel](https://vercel.com?utm_source=github&utm_medium=readme&utm_campaign=next-example):
[](https://vercel.com/new/git/external?repository-url=https://github.com/vercel/next.js/tree/canary/examples/hello-world&project-name=hello-world&repository-name=hello-world)
diff --git a/examples/i18n-routing/README.md b/examples/i18n-routing/README.md
index 2af5f145ad10b..38bbe207de1a8 100644
--- a/examples/i18n-routing/README.md
+++ b/examples/i18n-routing/README.md
@@ -6,7 +6,7 @@ For further documentation on this feature see the documentation [here](https://n
## Deploy your own
-Deploy the example using [Vercel](https://vercel.com):
+Deploy the example using [Vercel](https://vercel.com?utm_source=github&utm_medium=readme&utm_campaign=next-example):
[](https://vercel.com/new/git/external?repository-url=https://github.com/vercel/next.js/tree/canary/examples/i18n-routing&project-name=i18n-routing&repository-name=i18n-routing)
diff --git a/examples/image-component/README.md b/examples/image-component/README.md
index d1ddb65b8b72e..c44c4b139ebad 100644
--- a/examples/image-component/README.md
+++ b/examples/image-component/README.md
@@ -6,7 +6,7 @@ The index page ([`pages/index.js`](pages/index.js)) has a couple images, one int
## Deploy your own
-Deploy the example using [Vercel](https://vercel.com):
+Deploy the example using [Vercel](https://vercel.com?utm_source=github&utm_medium=readme&utm_campaign=next-example):
[](https://vercel.com/new/git/external?repository-url=https://github.com/vercel/next.js/tree/canary/examples/image-component&project-name=image-component&repository-name=image-component)
diff --git a/examples/layout-component/README.md b/examples/layout-component/README.md
index 1debb26dea5da..e9275efae9bf0 100644
--- a/examples/layout-component/README.md
+++ b/examples/layout-component/README.md
@@ -4,7 +4,7 @@ This example shows a very common use case when building websites where you need
## Deploy your own
-Deploy the example using [Vercel](https://vercel.com):
+Deploy the example using [Vercel](https://vercel.com?utm_source=github&utm_medium=readme&utm_campaign=next-example):
[](https://vercel.com/new/git/external?repository-url=https://github.com/vercel/next.js/tree/canary/examples/layout-component&project-name=layout-component&repository-name=layout-component)
diff --git a/examples/nested-components/README.md b/examples/nested-components/README.md
index ab1a3e75db488..8aa90224ae367 100644
--- a/examples/nested-components/README.md
+++ b/examples/nested-components/README.md
@@ -4,7 +4,7 @@ Taking advantage of the composable nature of React components we can modularize
## Deploy your own
-Deploy the example using [Vercel](https://vercel.com):
+Deploy the example using [Vercel](https://vercel.com?utm_source=github&utm_medium=readme&utm_campaign=next-example):
[](https://vercel.com/new/git/external?repository-url=https://github.com/vercel/next.js/tree/canary/examples/nested-components&project-name=nested-components&repository-name=nested-components)
diff --git a/examples/progressive-render/README.md b/examples/progressive-render/README.md
index 999f9bbd5da24..cec6253c342d3 100644
--- a/examples/progressive-render/README.md
+++ b/examples/progressive-render/README.md
@@ -18,7 +18,7 @@ This example features:
## Deploy your own
-Deploy the example using [Vercel](https://vercel.com):
+Deploy the example using [Vercel](https://vercel.com?utm_source=github&utm_medium=readme&utm_campaign=next-example):
[](https://vercel.com/new/git/external?repository-url=https://github.com/vercel/next.js/tree/canary/examples/progressive-render&project-name=progressive-render&repository-name=progressive-render)
diff --git a/examples/progressive-web-app/README.md b/examples/progressive-web-app/README.md
index b8a4c69463cb1..1358917e92f65 100644
--- a/examples/progressive-web-app/README.md
+++ b/examples/progressive-web-app/README.md
@@ -4,7 +4,7 @@ This example uses [`next-pwa`](https://github.com/shadowwalker/next-pwa) to crea
## Deploy your own
-Deploy the example using [Vercel](https://vercel.com):
+Deploy the example using [Vercel](https://vercel.com?utm_source=github&utm_medium=readme&utm_campaign=next-example):
[](https://vercel.com/new/git/external?repository-url=https://github.com/vercel/next.js/tree/canary/examples/progressive-web-app&project-name=progressive-web-app&repository-name=progressive-web-app)
diff --git a/examples/redirects/README.md b/examples/redirects/README.md
index 9cddc0fdd8314..88cbcfe10c7b8 100644
--- a/examples/redirects/README.md
+++ b/examples/redirects/README.md
@@ -6,7 +6,7 @@ The index page ([`pages/index.js`](pages/index.js)) has a list of links that mat
## Deploy your own
-Deploy the example using [Vercel](https://vercel.com):
+Deploy the example using [Vercel](https://vercel.com?utm_source=github&utm_medium=readme&utm_campaign=next-example):
[](https://vercel.com/new/git/external?repository-url=https://github.com/vercel/next.js/tree/canary/examples/redirects&project-name=redirects&repository-name=redirects)
diff --git a/examples/rewrites/README.md b/examples/rewrites/README.md
index f48630cdec7ad..5281816b25131 100644
--- a/examples/rewrites/README.md
+++ b/examples/rewrites/README.md
@@ -6,7 +6,7 @@ The index page ([`pages/index.js`](pages/index.js)) has a list of links that mat
## Deploy your own
-Deploy the example using [Vercel](https://vercel.com):
+Deploy the example using [Vercel](https://vercel.com?utm_source=github&utm_medium=readme&utm_campaign=next-example):
[](https://vercel.com/new/git/external?repository-url=https://github.com/vercel/next.js/tree/canary/examples/rewrites&project-name=rewrites&repository-name=rewrites)
diff --git a/examples/svg-components/README.md b/examples/svg-components/README.md
index bd155b7ea0f8f..bdb18158b40e2 100644
--- a/examples/svg-components/README.md
+++ b/examples/svg-components/README.md
@@ -4,7 +4,7 @@ This example uses a custom `.babelrc` to add support for importing `.svg` files
## Deploy your own
-Deploy the example using [Vercel](https://vercel.com):
+Deploy the example using [Vercel](https://vercel.com?utm_source=github&utm_medium=readme&utm_campaign=next-example):
[](https://vercel.com/new/git/external?repository-url=https://github.com/vercel/next.js/tree/canary/examples/svg-components&project-name=svg-components&repository-name=svg-components)
diff --git a/examples/using-router/README.md b/examples/using-router/README.md
index 569ef5cae2bdb..cc3ab1c3976a1 100644
--- a/examples/using-router/README.md
+++ b/examples/using-router/README.md
@@ -7,7 +7,7 @@ This example features:
## Deploy your own
-Deploy the example using [Vercel](https://vercel.com):
+Deploy the example using [Vercel](https://vercel.com?utm_source=github&utm_medium=readme&utm_campaign=next-example):
[](https://vercel.com/new/git/external?repository-url=https://github.com/vercel/next.js/tree/canary/examples/using-router&project-name=using-router&repository-name=using-router)
diff --git a/examples/with-absolute-imports/README.md b/examples/with-absolute-imports/README.md
index 166e4497254d7..c32e4eca78479 100644
--- a/examples/with-absolute-imports/README.md
+++ b/examples/with-absolute-imports/README.md
@@ -4,7 +4,7 @@ This example shows how to configure Babel to have absolute imports instead of re
## Deploy your own
-Deploy the example using [Vercel](https://vercel.com):
+Deploy the example using [Vercel](https://vercel.com?utm_source=github&utm_medium=readme&utm_campaign=next-example):
[](https://vercel.com/new/git/external?repository-url=https://github.com/vercel/next.js/tree/canary/examples/with-absolute-imports&project-name=with-absolute-imports&repository-name=with-absolute-imports)
diff --git a/examples/with-ant-design-less/README.md b/examples/with-ant-design-less/README.md
index 41e3cd622f013..6241f2458afd0 100644
--- a/examples/with-ant-design-less/README.md
+++ b/examples/with-ant-design-less/README.md
@@ -4,7 +4,7 @@ This example shows how to use Next.js along with [Ant Design of React](https://a
## Deploy your own
-Deploy the example using [Vercel](https://vercel.com):
+Deploy the example using [Vercel](https://vercel.com?utm_source=github&utm_medium=readme&utm_campaign=next-example):
[](https://vercel.com/new/git/external?repository-url=https://github.com/vercel/next.js/tree/canary/examples/with-ant-design-less&project-name=with-ant-design-less&repository-name=with-ant-design-less)
diff --git a/examples/with-ant-design-mobile/README.md b/examples/with-ant-design-mobile/README.md
index 29cd64b0fd8f4..be8f9a0dff82b 100644
--- a/examples/with-ant-design-mobile/README.md
+++ b/examples/with-ant-design-mobile/README.md
@@ -4,7 +4,7 @@ This example features how you use [antd-mobile](https://github.com/ant-design/an
## Deploy your own
-Deploy the example using [Vercel](https://vercel.com):
+Deploy the example using [Vercel](https://vercel.com?utm_source=github&utm_medium=readme&utm_campaign=next-example):
[](https://vercel.com/new/git/external?repository-url=https://github.com/vercel/next.js/tree/canary/examples/with-ant-design-mobile&project-name=with-ant-design-mobile&repository-name=with-ant-design-mobile)
diff --git a/examples/with-ant-design-pro-layout-less/README.md b/examples/with-ant-design-pro-layout-less/README.md
index fdf3e1a0a86d3..ff6d5fd94eb2e 100644
--- a/examples/with-ant-design-pro-layout-less/README.md
+++ b/examples/with-ant-design-pro-layout-less/README.md
@@ -4,7 +4,7 @@ This example shows how to use Next.js along with [Ant Design Pro Layout](https:/
## Deploy your own
-Deploy the example using [Vercel](https://vercel.com):
+Deploy the example using [Vercel](https://vercel.com?utm_source=github&utm_medium=readme&utm_campaign=next-example):
[](https://vercel.com/new/git/external?repository-url=https://github.com/vercel/next.js/tree/canary/examples/with-ant-design-pro-layout-less&project-name=with-ant-design-pro-layout-less&repository-name=with-ant-design-pro-layout-less)
diff --git a/examples/with-ant-design/README.md b/examples/with-ant-design/README.md
index ae996f0195a68..048316057ec35 100644
--- a/examples/with-ant-design/README.md
+++ b/examples/with-ant-design/README.md
@@ -4,7 +4,7 @@ This example shows how to use Next.js along with [Ant Design of React](https://a
## Deploy your own
-Deploy the example using [Vercel](https://vercel.com):
+Deploy the example using [Vercel](https://vercel.com?utm_source=github&utm_medium=readme&utm_campaign=next-example):
[](https://vercel.com/new/git/external?repository-url=https://github.com/vercel/next.js/tree/canary/examples/with-ant-design&project-name=with-ant-design&repository-name=with-ant-design)
diff --git a/examples/with-aphrodite/README.md b/examples/with-aphrodite/README.md
index 0f6cbd76c4e5a..b847040e8cd06 100644
--- a/examples/with-aphrodite/README.md
+++ b/examples/with-aphrodite/README.md
@@ -6,7 +6,7 @@ For this purpose we are extending the `` and injecting the server si
## Deploy your own
-Deploy the example using [Vercel](https://vercel.com):
+Deploy the example using [Vercel](https://vercel.com?utm_source=github&utm_medium=readme&utm_campaign=next-example):
[](https://vercel.com/new/git/external?repository-url=https://github.com/vercel/next.js/tree/canary/examples/with-aphrodite&project-name=with-aphrodite&repository-name=with-aphrodite)
diff --git a/examples/with-apollo-and-redux/README.md b/examples/with-apollo-and-redux/README.md
index d2bd99b9dac83..91dd69a7e2b93 100644
--- a/examples/with-apollo-and-redux/README.md
+++ b/examples/with-apollo-and-redux/README.md
@@ -8,7 +8,7 @@ To inspect the GraphQL API, use its [web IDE](https://nextjs-graphql-with-prisma
## Deploy your own
-Deploy the example using [Vercel](https://vercel.com):
+Deploy the example using [Vercel](https://vercel.com?utm_source=github&utm_medium=readme&utm_campaign=next-example):
[](https://vercel.com/new/git/external?repository-url=https://github.com/vercel/next.js/tree/canary/examples/with-apollo-and-redux&project-name=with-apollo-and-redux&repository-name=with-apollo-and-redux)
diff --git a/examples/with-apollo/README.md b/examples/with-apollo/README.md
index e7f44cda6724f..f11e9ee220214 100644
--- a/examples/with-apollo/README.md
+++ b/examples/with-apollo/README.md
@@ -12,7 +12,7 @@ This example relies on [Prisma + Nexus](https://github.com/prisma-labs/nextjs-gr
## Deploy your own
-Deploy the example using [Vercel](https://vercel.com):
+Deploy the example using [Vercel](https://vercel.com?utm_source=github&utm_medium=readme&utm_campaign=next-example):
[](https://vercel.com/new/git/external?repository-url=https://github.com/vercel/next.js/tree/canary/examples/with-apollo&project-name=with-apollo&repository-name=with-apollo)
diff --git a/examples/with-app-layout/README.md b/examples/with-app-layout/README.md
index 91dfec716778e..2e16bdd1f251e 100644
--- a/examples/with-app-layout/README.md
+++ b/examples/with-app-layout/README.md
@@ -4,7 +4,7 @@ Shows how to use \_app.js to implement a global layout for all pages.
## Deploy your own
-Deploy the example using [Vercel](https://vercel.com):
+Deploy the example using [Vercel](https://vercel.com?utm_source=github&utm_medium=readme&utm_campaign=next-example):
[](https://vercel.com/new/git/external?repository-url=https://github.com/vercel/next.js/tree/canary/examples/with-app-layout&project-name=with-app-layout&repository-name=with-app-layout)
diff --git a/examples/with-astroturf/README.md b/examples/with-astroturf/README.md
index 819b9cafc4e6d..d20ea9e350e5f 100644
--- a/examples/with-astroturf/README.md
+++ b/examples/with-astroturf/README.md
@@ -4,7 +4,7 @@ This example features how to use [astroturf](https://github.com/4Catalyzer/astro
## Deploy your own
-Deploy the example using [Vercel](https://vercel.com):
+Deploy the example using [Vercel](https://vercel.com?utm_source=github&utm_medium=readme&utm_campaign=next-example):
[](https://vercel.com/new/git/external?repository-url=https://github.com/vercel/next.js/tree/canary/examples/with-astroturf&project-name=with-astroturf&repository-name=with-astroturf)
diff --git a/examples/with-babel-macros/README.md b/examples/with-babel-macros/README.md
index 05e16d013dc96..fee17d90277d5 100644
--- a/examples/with-babel-macros/README.md
+++ b/examples/with-babel-macros/README.md
@@ -4,7 +4,7 @@ This example features how to configure and use [`babel-macros`](https://github.c
## Deploy your own
-Deploy the example using [Vercel](https://vercel.com):
+Deploy the example using [Vercel](https://vercel.com?utm_source=github&utm_medium=readme&utm_campaign=next-example):
[](https://vercel.com/new/git/external?repository-url=https://github.com/vercel/next.js/tree/canary/examples/with-babel-macros&project-name=with-babel-macros&repository-name=with-babel-macros)
diff --git a/examples/with-carbon-components/README.md b/examples/with-carbon-components/README.md
index 109e9ae2db0b0..af10357c70ee0 100644
--- a/examples/with-carbon-components/README.md
+++ b/examples/with-carbon-components/README.md
@@ -6,7 +6,7 @@ Create your own theme with Carbon Design System's [theming tools](https://themes
## Deploy your own
-Deploy the example using [Vercel](https://vercel.com):
+Deploy the example using [Vercel](https://vercel.com?utm_source=github&utm_medium=readme&utm_campaign=next-example):
[](https://vercel.com/new/git/external?repository-url=https://github.com/vercel/next.js/tree/canary/examples/with-carbon-components&project-name=with-carbon-components&repository-name=with-carbon-components)
diff --git a/examples/with-cerebral/README.md b/examples/with-cerebral/README.md
index c239d29f570ce..42e068afeef18 100644
--- a/examples/with-cerebral/README.md
+++ b/examples/with-cerebral/README.md
@@ -36,7 +36,7 @@ function getUser() {
## Deploy your own
-Deploy the example using [Vercel](https://vercel.com):
+Deploy the example using [Vercel](https://vercel.com?utm_source=github&utm_medium=readme&utm_campaign=next-example):
[](https://vercel.com/new/git/external?repository-url=https://github.com/vercel/next.js/tree/canary/examples/with-cerebral&project-name=with-cerebral&repository-name=with-cerebral)
diff --git a/examples/with-chakra-ui-typescript/README.md b/examples/with-chakra-ui-typescript/README.md
index 90ec334343a23..e2966eb95d563 100644
--- a/examples/with-chakra-ui-typescript/README.md
+++ b/examples/with-chakra-ui-typescript/README.md
@@ -8,7 +8,7 @@ We are connecting the Next.js `_app.js` with `chakra-ui`'s Provider and theme so
## Deploy your own
-Deploy the example using [Vercel](https://vercel.com):
+Deploy the example using [Vercel](https://vercel.com?utm_source=github&utm_medium=readme&utm_campaign=next-example):
[](https://vercel.com/new/git/external?repository-url=https://github.com/vercel/next.js/tree/canary/examples/with-chakra-ui-typescript&project-name=with-chakra-ui-typescript&repository-name=with-chakra-ui-typescript)
diff --git a/examples/with-chakra-ui/README.md b/examples/with-chakra-ui/README.md
index b46ca6f14eee3..4c62d18b49058 100644
--- a/examples/with-chakra-ui/README.md
+++ b/examples/with-chakra-ui/README.md
@@ -6,7 +6,7 @@ We are connecting the Next.js `_app.js` with `chakra-ui`'s Theme and ColorMode c
## Deploy your own
-Deploy the example using [Vercel](https://vercel.com):
+Deploy the example using [Vercel](https://vercel.com?utm_source=github&utm_medium=readme&utm_campaign=next-example):
[](https://vercel.com/new/git/external?repository-url=https://github.com/vercel/next.js/tree/canary/examples/with-chakra-ui&project-name=with-chakra-ui&repository-name=with-chakra-ui)
diff --git a/examples/with-context-api/README.md b/examples/with-context-api/README.md
index 01b4b176417ec..65ca4dfdfaa9a 100644
--- a/examples/with-context-api/README.md
+++ b/examples/with-context-api/README.md
@@ -12,7 +12,7 @@ The `pages/about.js` shows how to pass an increment value from the about page in
## Deploy your own
-Deploy the example using [Vercel](https://vercel.com):
+Deploy the example using [Vercel](https://vercel.com?utm_source=github&utm_medium=readme&utm_campaign=next-example):
[](https://vercel.com/new/git/external?repository-url=https://github.com/vercel/next.js/tree/canary/examples/with-context-api&project-name=with-context-api&repository-name=with-context-api)
diff --git a/examples/with-cssed/README.md b/examples/with-cssed/README.md
index cf6f512616122..5e2dedfb9052d 100644
--- a/examples/with-cssed/README.md
+++ b/examples/with-cssed/README.md
@@ -6,7 +6,7 @@ We are creating `div` element with local scoped styles. The styles includes the
## Deploy your own
-Deploy the example using [Vercel](https://vercel.com):
+Deploy the example using [Vercel](https://vercel.com?utm_source=github&utm_medium=readme&utm_campaign=next-example):
[](https://vercel.com/new/git/external?repository-url=https://github.com/vercel/next.js/tree/canary/examples/with-cssed&project-name=with-cssed&repository-name=with-cssed)
diff --git a/examples/with-custom-babel-config/README.md b/examples/with-custom-babel-config/README.md
index d2c8e6d2acd00..01f05f36bc9df 100644
--- a/examples/with-custom-babel-config/README.md
+++ b/examples/with-custom-babel-config/README.md
@@ -10,7 +10,7 @@ This example features:
## Deploy your own
-Deploy the example using [Vercel](https://vercel.com):
+Deploy the example using [Vercel](https://vercel.com?utm_source=github&utm_medium=readme&utm_campaign=next-example):
[](https://vercel.com/new/git/external?repository-url=https://github.com/vercel/next.js/tree/canary/examples/with-custom-babel-config&project-name=with-custom-babel-config&repository-name=with-custom-babel-config)
diff --git a/examples/with-cxs/README.md b/examples/with-cxs/README.md
index 9989d9f0096c6..dda8a6151269b 100644
--- a/examples/with-cxs/README.md
+++ b/examples/with-cxs/README.md
@@ -6,7 +6,7 @@ For this purpose we are extending the `` and injecting the server si
## Deploy your own
-Deploy the example using [Vercel](https://vercel.com):
+Deploy the example using [Vercel](https://vercel.com?utm_source=github&utm_medium=readme&utm_campaign=next-example):
[](https://vercel.com/new/git/external?repository-url=https://github.com/vercel/next.js/tree/canary/examples/with-cxs&project-name=with-cxs&repository-name=with-cxs)
diff --git a/examples/with-draft-js/README.md b/examples/with-draft-js/README.md
index 49b9ce1003a04..a445cc97e442b 100644
--- a/examples/with-draft-js/README.md
+++ b/examples/with-draft-js/README.md
@@ -6,7 +6,7 @@ This example aims to provide a fully customizable example of the famous medium e
## Deploy your own
-Deploy the example using [Vercel](https://vercel.com):
+Deploy the example using [Vercel](https://vercel.com?utm_source=github&utm_medium=readme&utm_campaign=next-example):
[](https://vercel.com/new/git/external?repository-url=https://github.com/vercel/next.js/tree/canary/examples/with-draft-js&project-name=with-draft-js&repository-name=with-draft-js)
diff --git a/examples/with-dynamic-app-layout/README.md b/examples/with-dynamic-app-layout/README.md
index ff8cb359ff139..d23ec233c4f42 100644
--- a/examples/with-dynamic-app-layout/README.md
+++ b/examples/with-dynamic-app-layout/README.md
@@ -4,7 +4,7 @@ Shows how to use `pages/_app.js` to implement _dynamic_ layouts for pages. This
## Deploy your own
-Deploy the example using [Vercel](https://vercel.com):
+Deploy the example using [Vercel](https://vercel.com?utm_source=github&utm_medium=readme&utm_campaign=next-example):
[](https://vercel.com/new/git/external?repository-url=https://github.com/vercel/next.js/tree/canary/examples/with-dynamic-app-layout&project-name=with-dynamic-app-layout&repository-name=with-dynamic-app-layout)
diff --git a/examples/with-dynamic-import/README.md b/examples/with-dynamic-import/README.md
index 6a16b0e409044..0360408bc002e 100644
--- a/examples/with-dynamic-import/README.md
+++ b/examples/with-dynamic-import/README.md
@@ -4,7 +4,7 @@ This examples shows how to dynamically import modules via [`import()`](https://g
## Deploy your own
-Deploy the example using [Vercel](https://vercel.com):
+Deploy the example using [Vercel](https://vercel.com?utm_source=github&utm_medium=readme&utm_campaign=next-example):
[](https://vercel.com/new/git/external?repository-url=https://github.com/vercel/next.js/tree/canary/examples/with-dynamic-import&project-name=with-dynamic-import&repository-name=with-dynamic-import)
diff --git a/examples/with-emotion/README.md b/examples/with-emotion/README.md
index 09f34a7215b0b..a46123c4f027c 100644
--- a/examples/with-emotion/README.md
+++ b/examples/with-emotion/README.md
@@ -8,7 +8,7 @@ and [@emotion/styled](https://github.com/emotion-js/emotion/tree/master/packages
## Deploy your own
-Deploy the example using [Vercel](https://vercel.com):
+Deploy the example using [Vercel](https://vercel.com?utm_source=github&utm_medium=readme&utm_campaign=next-example):
[](https://vercel.com/new/git/external?repository-url=https://github.com/vercel/next.js/tree/canary/examples/with-emotion&project-name=with-emotion&repository-name=with-emotion)
diff --git a/examples/with-env-from-next-config-js/README.md b/examples/with-env-from-next-config-js/README.md
index bcb6873a7cfbb..0b08c49b5108b 100644
--- a/examples/with-env-from-next-config-js/README.md
+++ b/examples/with-env-from-next-config-js/README.md
@@ -14,7 +14,7 @@ View the docs on [`next.config.js`](https://nextjs.org/docs/api-reference/next.c
## Deploy your own
-Deploy the example using [Vercel](https://vercel.com):
+Deploy the example using [Vercel](https://vercel.com?utm_source=github&utm_medium=readme&utm_campaign=next-example):
[](https://vercel.com/new/git/external?repository-url=https://github.com/vercel/next.js/tree/canary/examples/with-env-from-next-config-js&project-name=with-env-from-next-config-js&repository-name=with-env-from-next-config-js)
diff --git a/examples/with-expo-typescript/README.md b/examples/with-expo-typescript/README.md
index 327b4cba3ab49..667d53bd1f5f1 100644
--- a/examples/with-expo-typescript/README.md
+++ b/examples/with-expo-typescript/README.md
@@ -20,7 +20,7 @@ This is a starter project for creating universal React apps with Next.js, Expo,
## Deploy your own
-Deploy the example using [Vercel](https://vercel.com) (web only):
+Deploy the example using [Vercel](https://vercel.com?utm_source=github&utm_medium=readme&utm_campaign=next-example) (web only):
[](https://vercel.com/new/git/external?repository-url=https://github.com/vercel/next.js/tree/canary/examples/with-expo&project-name=with-expo&repository-name=with-expo)
diff --git a/examples/with-expo/README.md b/examples/with-expo/README.md
index 37598f1419e4c..898131a8a640f 100644
--- a/examples/with-expo/README.md
+++ b/examples/with-expo/README.md
@@ -20,7 +20,7 @@ This is a starter project for creating universal React apps with Next.js and Exp
## Deploy your own
-Deploy the example using [Vercel](https://vercel.com) (web only):
+Deploy the example using [Vercel](https://vercel.com?utm_source=github&utm_medium=readme&utm_campaign=next-example) (web only):
[](https://vercel.com/new/git/external?repository-url=https://github.com/vercel/next.js/tree/canary/examples/with-expo&project-name=with-expo&repository-name=with-expo)
diff --git a/examples/with-facebook-pixel/README.md b/examples/with-facebook-pixel/README.md
index d28c265058a56..fbc75d0f6bde6 100644
--- a/examples/with-facebook-pixel/README.md
+++ b/examples/with-facebook-pixel/README.md
@@ -4,7 +4,7 @@ This example shows how to use Next.js along with Facebook Pixel. A custom [\_doc
## Deploy your own
-Deploy the example using [Vercel](https://vercel.com):
+Deploy the example using [Vercel](https://vercel.com?utm_source=github&utm_medium=readme&utm_campaign=next-example):
[](https://vercel.com/new/git/external?repository-url=https://github.com/vercel/next.js/tree/canary/examples/with-facebook-pixel&project-name=with-facebook-pixel&repository-name=with-facebook-pixel)
diff --git a/examples/with-fela/README.md b/examples/with-fela/README.md
index 9eff75b0abcf7..f42ee2e5754e5 100755
--- a/examples/with-fela/README.md
+++ b/examples/with-fela/README.md
@@ -6,7 +6,7 @@ For this purpose we are extending the `` and injecting the server si
## Deploy your own
-Deploy the example using [Vercel](https://vercel.com):
+Deploy the example using [Vercel](https://vercel.com?utm_source=github&utm_medium=readme&utm_campaign=next-example):
[](https://vercel.com/new/git/external?repository-url=https://github.com/vercel/next.js/tree/canary/examples/with-fela&project-name=with-fela&repository-name=with-fela)
diff --git a/examples/with-filbert/README.md b/examples/with-filbert/README.md
index 59bb30f62b20c..b98156888bdd2 100644
--- a/examples/with-filbert/README.md
+++ b/examples/with-filbert/README.md
@@ -8,7 +8,7 @@ If you are running into any issues with this example, feel free to open-up an is
## Deploy your own
-Deploy the example using [Vercel](https://vercel.com):
+Deploy the example using [Vercel](https://vercel.com?utm_source=github&utm_medium=readme&utm_campaign=next-example):
[](https://vercel.com/new/git/external?repository-url=https://github.com/vercel/next.js/tree/canary/examples/with-filbert&project-name=with-filbert&repository-name=with-filbert)
diff --git a/examples/with-firebase/README.md b/examples/with-firebase/README.md
index a71a161ff4575..21f4d46385f54 100644
--- a/examples/with-firebase/README.md
+++ b/examples/with-firebase/README.md
@@ -8,7 +8,7 @@ The React Context API is used to provide user state.
## Deploy your own
-Deploy the example using [Vercel](https://vercel.com):
+Deploy the example using [Vercel](https://vercel.com?utm_source=github&utm_medium=readme&utm_campaign=next-example):
[](https://vercel.com/new/git/external?repository-url=https://github.com/vercel/next.js/tree/canary/examples/with-firebase&project-name=with-firebase&repository-name=with-firebase)
diff --git a/examples/with-flow/README.md b/examples/with-flow/README.md
index 7da827c646bd1..dd8146e33b72f 100644
--- a/examples/with-flow/README.md
+++ b/examples/with-flow/README.md
@@ -4,7 +4,7 @@ This example shows how you can use Flow, with the transform-flow-strip-types bab
## Deploy your own
-Deploy the example using [Vercel](https://vercel.com):
+Deploy the example using [Vercel](https://vercel.com?utm_source=github&utm_medium=readme&utm_campaign=next-example):
[](https://vercel.com/new/git/external?repository-url=https://github.com/vercel/next.js/tree/canary/examples/with-flow&project-name=with-flow&repository-name=with-flow)
diff --git a/examples/with-framer-motion/README.md b/examples/with-framer-motion/README.md
index f7d7d06be71b7..b37b05db09592 100644
--- a/examples/with-framer-motion/README.md
+++ b/examples/with-framer-motion/README.md
@@ -6,7 +6,7 @@ When using Next's `` component, you will likely want to [disable the defau
## Deploy your own
-Deploy the example using [Vercel](https://vercel.com):
+Deploy the example using [Vercel](https://vercel.com?utm_source=github&utm_medium=readme&utm_campaign=next-example):
[](https://vercel.com/new/git/external?repository-url=https://github.com/vercel/next.js/tree/canary/examples/with-framer-motion&project-name=with-framer-motion&repository-name=with-framer-motion)
diff --git a/examples/with-glamor/README.md b/examples/with-glamor/README.md
index bbc88c108914e..c09f9d12aa4e3 100644
--- a/examples/with-glamor/README.md
+++ b/examples/with-glamor/README.md
@@ -8,7 +8,7 @@ In this example a custom React.createElement is used. With the help of a babel p
## Deploy your own
-Deploy the example using [Vercel](https://vercel.com):
+Deploy the example using [Vercel](https://vercel.com?utm_source=github&utm_medium=readme&utm_campaign=next-example):
[](https://vercel.com/new/git/external?repository-url=https://github.com/vercel/next.js/tree/canary/examples/with-glamor&project-name=with-glamor&repository-name=with-glamor)
diff --git a/examples/with-google-analytics-amp/README.md b/examples/with-google-analytics-amp/README.md
index 86b6c9c9f0db4..8d7e8c6fa9c49 100644
--- a/examples/with-google-analytics-amp/README.md
+++ b/examples/with-google-analytics-amp/README.md
@@ -4,7 +4,7 @@ This example shows how to use [Next.js](https://github.com/vercel/next.js) along
## Deploy your own
-Deploy the example using [Vercel](https://vercel.com):
+Deploy the example using [Vercel](https://vercel.com?utm_source=github&utm_medium=readme&utm_campaign=next-example):
[](https://vercel.com/new/git/external?repository-url=https://github.com/vercel/next.js/tree/canary/examples/with-google-analytics-amp&project-name=with-google-analytics-amp&repository-name=with-google-analytics-amp)
diff --git a/examples/with-google-analytics/README.md b/examples/with-google-analytics/README.md
index 63ddbbf270fa3..c449f4ea08939 100644
--- a/examples/with-google-analytics/README.md
+++ b/examples/with-google-analytics/README.md
@@ -4,7 +4,7 @@ This example shows how to use [Next.js](https://github.com/vercel/next.js) along
## Deploy your own
-Deploy the example using [Vercel](https://vercel.com):
+Deploy the example using [Vercel](https://vercel.com?utm_source=github&utm_medium=readme&utm_campaign=next-example):
[](https://vercel.com/new/git/external?repository-url=https://github.com/vercel/next.js/tree/canary/examples/with-google-analytics&project-name=with-google-analytics&repository-name=with-google-analytics)
diff --git a/examples/with-google-tag-manager/README.md b/examples/with-google-tag-manager/README.md
index 9db702cdd0fd4..a0a459140d90a 100644
--- a/examples/with-google-tag-manager/README.md
+++ b/examples/with-google-tag-manager/README.md
@@ -4,7 +4,7 @@ This example shows how to use Next.js along with Google Tag Manager. [`pages/_do
## Deploy your own
-Deploy the example using [Vercel](https://vercel.com):
+Deploy the example using [Vercel](https://vercel.com?utm_source=github&utm_medium=readme&utm_campaign=next-example):
[](https://vercel.com/new/git/external?repository-url=https://github.com/vercel/next.js/tree/canary/examples/with-google-tag-manager&project-name=with-google-tag-manager&repository-name=with-google-tag-manager)
diff --git a/examples/with-graphql-faunadb/README.md b/examples/with-graphql-faunadb/README.md
index 98427292e7567..19c03688a499e 100644
--- a/examples/with-graphql-faunadb/README.md
+++ b/examples/with-graphql-faunadb/README.md
@@ -4,7 +4,7 @@ This simple Guestbook SPA example shows you how to use [FaunaDB's GraphQL endpoi
## Deploy your own
-Deploy the example using [Vercel](https://vercel.com):
+Deploy the example using [Vercel](https://vercel.com?utm_source=github&utm_medium=readme&utm_campaign=next-example):
[](https://vercel.com/new/git/external?repository-url=https://github.com/vercel/next.js/tree/canary/examples/with-graphql-faunadb&project-name=my-nextjs-guestbook&repository-name=my-nextjs-guestbook&env=NEXT_PUBLIC_FAUNADB_SECRET,NEXT_PUBLIC_FAUNADB_GRAPHQL_ENDPOINT&envDescription=Client%20secret%20and%20GraphQL%20endpoint%20needed%20for%20communicating%20with%20the%20live%20Fauna%20database&demo-title=Next.js%20Fauna%20Guestbook%20App&demo-description=A%20simple%20guestbook%20application%20built%20with%20Next.js%20and%20Fauna&demo-url=https%3A%2F%2Fnextjs-guestbook.vercel.app%2F)
diff --git a/examples/with-graphql-hooks/README.md b/examples/with-graphql-hooks/README.md
index 4f8dc9ce32a69..546696c9056d7 100644
--- a/examples/with-graphql-hooks/README.md
+++ b/examples/with-graphql-hooks/README.md
@@ -8,7 +8,7 @@ This started life as a copy of the `with-apollo` example. We then stripped out A
## Deploy your own
-Deploy the example using [Vercel](https://vercel.com):
+Deploy the example using [Vercel](https://vercel.com?utm_source=github&utm_medium=readme&utm_campaign=next-example):
[](https://vercel.com/new/git/external?repository-url=https://github.com/vercel/next.js/tree/canary/examples/with-graphql-hooks&project-name=with-graphql-hooks&repository-name=with-graphql-hooks)
diff --git a/examples/with-graphql-react/README.md b/examples/with-graphql-react/README.md
index 38b0ed7780d71..c7b0830916630 100644
--- a/examples/with-graphql-react/README.md
+++ b/examples/with-graphql-react/README.md
@@ -4,7 +4,7 @@
## Deploy your own
-Deploy the example using [Vercel](https://vercel.com):
+Deploy the example using [Vercel](https://vercel.com?utm_source=github&utm_medium=readme&utm_campaign=next-example):
[](https://vercel.com/new/git/external?repository-url=https://github.com/vercel/next.js/tree/canary/examples/with-graphql-react&project-name=with-graphql-react&repository-name=with-graphql-react)
diff --git a/examples/with-grommet/README.md b/examples/with-grommet/README.md
index 0d04ea115521f..28861444023d1 100644
--- a/examples/with-grommet/README.md
+++ b/examples/with-grommet/README.md
@@ -6,7 +6,7 @@ It works by extending the `` to enable server-side rendering of `sty
## Deploy your own
-Deploy the example using [Vercel](https://vercel.com):
+Deploy the example using [Vercel](https://vercel.com?utm_source=github&utm_medium=readme&utm_campaign=next-example):
[](https://vercel.com/new/git/external?repository-url=https://github.com/vercel/next.js/tree/canary/examples/with-grommet&project-name=with-grommet&repository-name=with-grommet)
diff --git a/examples/with-gsap/README.md b/examples/with-gsap/README.md
index e2b1d55bb3ecf..3dbb4c5f67ee5 100644
--- a/examples/with-gsap/README.md
+++ b/examples/with-gsap/README.md
@@ -4,7 +4,7 @@ This example features how to use [GSAP](https://greensock.com/gsap/) as the anim
## Deploy your own
-Deploy the example using [Vercel](https://vercel.com):
+Deploy the example using [Vercel](https://vercel.com?utm_source=github&utm_medium=readme&utm_campaign=next-example):
[](https://vercel.com/new/git/external?repository-url=https://github.com/vercel/next.js/tree/canary/examples/with-gsap&project-name=with-gsap&repository-name=with-gsap)
diff --git a/examples/with-i18n-rosetta/README.md b/examples/with-i18n-rosetta/README.md
index 9f821cb267ceb..fa8ae884c11af 100644
--- a/examples/with-i18n-rosetta/README.md
+++ b/examples/with-i18n-rosetta/README.md
@@ -6,7 +6,7 @@ In `next.config.js` you can configure the fallback language.
## Deploy your own
-Deploy the example using [Vercel](https://vercel.com):
+Deploy the example using [Vercel](https://vercel.com?utm_source=github&utm_medium=readme&utm_campaign=next-example):
[](https://vercel.com/new/git/external?repository-url=https://github.com/vercel/next.js/tree/canary/examples/with-i18n-rosetta&project-name=with-i18n-rosetta&repository-name=with-i18n-rosetta)
diff --git a/examples/with-ionic-typescript/README.md b/examples/with-ionic-typescript/README.md
index a1d5dc85d7ee2..a56e57f9a0321 100644
--- a/examples/with-ionic-typescript/README.md
+++ b/examples/with-ionic-typescript/README.md
@@ -4,7 +4,7 @@ Example app using Next.js with [Ionic](https://ionicframework.com/) and [TypeScr
## Deploy your own
-Deploy the example using [Vercel](https://vercel.com):
+Deploy the example using [Vercel](https://vercel.com?utm_source=github&utm_medium=readme&utm_campaign=next-example):
[](https://vercel.com/new/git/external?repository-url=https://github.com/vercel/next.js/tree/canary/examples/with-ionic-typescript&project-name=with-ionic-typescript&repository-name=with-ionic-typescript)
diff --git a/examples/with-iron-session/README.md b/examples/with-iron-session/README.md
index 0e449313b2e4c..20defce31db69 100644
--- a/examples/with-iron-session/README.md
+++ b/examples/with-iron-session/README.md
@@ -25,7 +25,7 @@ It uses current best practices for authentication in the Next.js ecosystem.
## Deploy your own
-Deploy the example using [Vercel](https://vercel.com):
+Deploy the example using [Vercel](https://vercel.com?utm_source=github&utm_medium=readme&utm_campaign=next-example):
[](https://vercel.com/new/git/external?repository-url=https://github.com/vercel/next.js/tree/canary/examples/with-iron-session&project-name=with-iron-session&repository-name=with-iron-session)
diff --git a/examples/with-kea/README.md b/examples/with-kea/README.md
index ef0c8d9d5fde4..cfb3e36766601 100644
--- a/examples/with-kea/README.md
+++ b/examples/with-kea/README.md
@@ -4,7 +4,7 @@ This example uses [kea](https://github.com/keajs/kea).
## Deploy your own
-Deploy the example using [Vercel](https://vercel.com):
+Deploy the example using [Vercel](https://vercel.com?utm_source=github&utm_medium=readme&utm_campaign=next-example):
[](https://vercel.com/new/git/external?repository-url=https://github.com/vercel/next.js/tree/canary/examples/with-kea&project-name=with-kea&repository-name=with-kea)
diff --git a/examples/with-linaria/README.md b/examples/with-linaria/README.md
index bab1cfdce9064..61294975b32d3 100644
--- a/examples/with-linaria/README.md
+++ b/examples/with-linaria/README.md
@@ -6,7 +6,7 @@ We are creating three `div` elements with custom styles being shared across the
## Deploy your own
-Deploy the example using [Vercel](https://vercel.com):
+Deploy the example using [Vercel](https://vercel.com?utm_source=github&utm_medium=readme&utm_campaign=next-example):
[](https://vercel.com/new/git/external?repository-url=https://github.com/vercel/next.js/tree/canary/examples/with-linaria&project-name=with-linaria&repository-name=with-linaria)
diff --git a/examples/with-lingui/README.md b/examples/with-lingui/README.md
index 7c0082e337417..4111f52697f15 100644
--- a/examples/with-lingui/README.md
+++ b/examples/with-lingui/README.md
@@ -8,7 +8,7 @@ The example also uses a Higher order Component which can be added to all pages w
## Deploy your own
-Deploy the example using [Vercel](https://vercel.com):
+Deploy the example using [Vercel](https://vercel.com?utm_source=github&utm_medium=readme&utm_campaign=next-example):
[](https://vercel.com/new/git/external?repository-url=https://github.com/vercel/next.js/tree/canary/examples/with-lingui&project-name=with-lingui&repository-name=with-lingui)
diff --git a/examples/with-loading/README.md b/examples/with-loading/README.md
index 7598f4e7be1c2..5e3c0fd4d4dad 100644
--- a/examples/with-loading/README.md
+++ b/examples/with-loading/README.md
@@ -12,7 +12,7 @@ It features:
## Deploy your own
-Deploy the example using [Vercel](https://vercel.com):
+Deploy the example using [Vercel](https://vercel.com?utm_source=github&utm_medium=readme&utm_campaign=next-example):
[](https://vercel.com/new/git/external?repository-url=https://github.com/vercel/next.js/tree/canary/examples/with-loading&project-name=with-loading&repository-name=with-loading)
diff --git a/examples/with-mdbreact/README.md b/examples/with-mdbreact/README.md
index 898c4f0bd1cbe..979ca6d954e6f 100644
--- a/examples/with-mdbreact/README.md
+++ b/examples/with-mdbreact/README.md
@@ -4,7 +4,7 @@ This example shows how to use [MDBReact](https://mdbootstrap.com/docs/react) wit
## Deploy your own
-Deploy the example using [Vercel](https://vercel.com):
+Deploy the example using [Vercel](https://vercel.com?utm_source=github&utm_medium=readme&utm_campaign=next-example):
[](https://vercel.com/new/git/external?repository-url=https://github.com/vercel/next.js/tree/canary/examples/with-mdbreact&project-name=with-mdbreact&repository-name=with-mdbreact)
diff --git a/examples/with-mdx-remote/README.md b/examples/with-mdx-remote/README.md
index 937879aee4301..dacd0d03467f6 100644
--- a/examples/with-mdx-remote/README.md
+++ b/examples/with-mdx-remote/README.md
@@ -8,7 +8,7 @@ Since `next-remote-watch` uses undocumented Next.js APIs, it doesn't replace the
## Deploy your own
-Deploy the example using [Vercel](https://vercel.com):
+Deploy the example using [Vercel](https://vercel.com?utm_source=github&utm_medium=readme&utm_campaign=next-example):
[](https://vercel.com/new/git/external?repository-url=https://github.com/vercel/next.js/tree/canary/examples/with-mdx-remote&project-name=with-mdx-remote&repository-name=with-mdx-remote)
diff --git a/examples/with-mdx/README.md b/examples/with-mdx/README.md
index cea3de3fe656a..5544468ae2c3d 100644
--- a/examples/with-mdx/README.md
+++ b/examples/with-mdx/README.md
@@ -4,7 +4,7 @@ This example shows using [MDX](https://github.com/mdx-js/mdx) as top level pages
## Deploy your own
-Deploy the example using [Vercel](https://vercel.com):
+Deploy the example using [Vercel](https://vercel.com?utm_source=github&utm_medium=readme&utm_campaign=next-example):
[](https://vercel.com/new/git/external?repository-url=https://github.com/vercel/next.js/tree/canary/examples/with-mdx&project-name=with-mdx&repository-name=with-mdx)
diff --git a/examples/with-mobx-react-lite/README.md b/examples/with-mobx-react-lite/README.md
index d55b829152583..3ba0f7da966f3 100644
--- a/examples/with-mobx-react-lite/README.md
+++ b/examples/with-mobx-react-lite/README.md
@@ -18,7 +18,7 @@ Both components are using a custom hook `useStore` to pull in the `Store` from t
## Deploy your own
-Deploy the example using [Vercel](https://vercel.com):
+Deploy the example using [Vercel](https://vercel.com?utm_source=github&utm_medium=readme&utm_campaign=next-example):
[](https://vercel.com/new/git/external?repository-url=https://github.com/vercel/next.js/tree/canary/examples/with-mobx-react-lite&project-name=with-mobx-react-lite&repository-name=with-mobx-react-lite)
diff --git a/examples/with-mobx-state-tree-typescript/README.md b/examples/with-mobx-state-tree-typescript/README.md
index 08df6af0058e9..2b9925908edaf 100644
--- a/examples/with-mobx-state-tree-typescript/README.md
+++ b/examples/with-mobx-state-tree-typescript/README.md
@@ -12,7 +12,7 @@ The clock, under `components/Clock.js`, has access to the state using the `injec
## Deploy your own
-Deploy the example using [Vercel](https://vercel.com):
+Deploy the example using [Vercel](https://vercel.com?utm_source=github&utm_medium=readme&utm_campaign=next-example):
[](https://vercel.com/new/git/external?repository-url=https://github.com/vercel/next.js/tree/canary/examples/with-mobx-state-tree-typescript&project-name=with-mobx-state-tree-typescript&repository-name=with-mobx-state-tree-typescript)
diff --git a/examples/with-mobx-state-tree/README.md b/examples/with-mobx-state-tree/README.md
index 9c1ea92da1277..a81246eda93ef 100644
--- a/examples/with-mobx-state-tree/README.md
+++ b/examples/with-mobx-state-tree/README.md
@@ -12,7 +12,7 @@ The clock, under `components/Clock.js`, has access to the state using the `injec
## Deploy your own
-Deploy the example using [Vercel](https://vercel.com):
+Deploy the example using [Vercel](https://vercel.com?utm_source=github&utm_medium=readme&utm_campaign=next-example):
[](https://vercel.com/new/git/external?repository-url=https://github.com/vercel/next.js/tree/canary/examples/with-mobx-state-tree&project-name=with-mobx-state-tree&repository-name=with-mobx-state-tree)
diff --git a/examples/with-mobx/README.md b/examples/with-mobx/README.md
index c507638b358e6..fcd4b8934385f 100644
--- a/examples/with-mobx/README.md
+++ b/examples/with-mobx/README.md
@@ -12,7 +12,7 @@ The clock, under `components/Clock.js`, has access to the state using the `injec
## Deploy your own
-Deploy the example using [Vercel](https://vercel.com):
+Deploy the example using [Vercel](https://vercel.com?utm_source=github&utm_medium=readme&utm_campaign=next-example):
[](https://vercel.com/new/git/external?repository-url=https://github.com/vercel/next.js/tree/canary/examples/with-mobx&project-name=with-mobx&repository-name=with-mobx)
diff --git a/examples/with-monaco-editor/README.md b/examples/with-monaco-editor/README.md
index da8c61525e616..a6c70550d471f 100644
--- a/examples/with-monaco-editor/README.md
+++ b/examples/with-monaco-editor/README.md
@@ -5,7 +5,7 @@ This example adds support for [Monaco Editor](https://github.com/Microsoft/monac
## Deploy your own
-Deploy the example using [Vercel](https://vercel.com):
+Deploy the example using [Vercel](https://vercel.com?utm_source=github&utm_medium=readme&utm_campaign=next-example):
[](https://vercel.com/new/git/external?repository-url=https://github.com/vercel/next.js/tree/canary/examples/with-monaco-editor&project-name=with-monaco-editor&repository-name=with-monaco-editor)
diff --git a/examples/with-mongodb-mongoose/README.md b/examples/with-mongodb-mongoose/README.md
index f1d82bcbc61ed..a8a67e25e8dcf 100644
--- a/examples/with-mongodb-mongoose/README.md
+++ b/examples/with-mongodb-mongoose/README.md
@@ -6,7 +6,7 @@ This example shows how you can use a MongoDB database to support your Next.js ap
## Deploy your own
-Deploy the example using [Vercel](https://vercel.com):
+Deploy the example using [Vercel](https://vercel.com?utm_source=github&utm_medium=readme&utm_campaign=next-example):
[](https://vercel.com/new/git/external?repository-url=https://github.com/vercel/next.js/tree/canary/examples/with-mongodb-mongoose&project-name=with-mongodb-mongoose&repository-name=with-mongodb-mongoose)
diff --git a/examples/with-mqtt-js/README.md b/examples/with-mqtt-js/README.md
index 8be25489d84c2..ecd77590b1182 100644
--- a/examples/with-mqtt-js/README.md
+++ b/examples/with-mqtt-js/README.md
@@ -4,7 +4,7 @@ This example shows how to use [MQTT.js](https://github.com/mqttjs/MQTT.js) with
## Deploy your own
-Deploy the example using [Vercel](https://vercel.com):
+Deploy the example using [Vercel](https://vercel.com?utm_source=github&utm_medium=readme&utm_campaign=next-example):
[](https://vercel.com/new/git/external?repository-url=https://github.com/vercel/next.js/tree/canary/examples/with-mqtt-js&project-name=with-mqtt-js&repository-name=with-mqtt-js)
diff --git a/examples/with-msw/README.md b/examples/with-msw/README.md
index e1b9383480866..dd13738eac599 100644
--- a/examples/with-msw/README.md
+++ b/examples/with-msw/README.md
@@ -12,7 +12,7 @@ Mocking is enabled using the `NEXT_PUBLIC_API_MOCKING` environment variable. By
## Deploy your own
-Deploy the example using [Vercel](https://vercel.com):
+Deploy the example using [Vercel](https://vercel.com?utm_source=github&utm_medium=readme&utm_campaign=next-example):
[](https://vercel.com/new/git/external?repository-url=https://github.com/vercel/next.js/tree/canary/examples/with-msw&project-name=with-msw&repository-name=with-msw)
diff --git a/examples/with-next-css/README.md b/examples/with-next-css/README.md
index e993ab5430393..03db91557a14d 100644
--- a/examples/with-next-css/README.md
+++ b/examples/with-next-css/README.md
@@ -4,7 +4,7 @@ This example demonstrates how to use Next.js' built-in CSS imports and CSS modul
## Deploy your own
-Deploy the example using [Vercel](https://vercel.com):
+Deploy the example using [Vercel](https://vercel.com?utm_source=github&utm_medium=readme&utm_campaign=next-example):
[](https://vercel.com/new/git/external?repository-url=https://github.com/vercel/next.js/tree/canary/examples/with-next-css&project-name=with-next-css&repository-name=with-next-css)
diff --git a/examples/with-next-less/README.md b/examples/with-next-less/README.md
index 527aec36e3199..40b19e1250cc7 100644
--- a/examples/with-next-less/README.md
+++ b/examples/with-next-less/README.md
@@ -6,7 +6,7 @@ It includes patterns for with and without CSS Modules, with PostCSS and with add
## Deploy your own
-Deploy the example using [Vercel](https://vercel.com):
+Deploy the example using [Vercel](https://vercel.com?utm_source=github&utm_medium=readme&utm_campaign=next-example):
[](https://vercel.com/new/git/external?repository-url=https://github.com/vercel/next.js/tree/canary/examples/with-next-less&project-name=with-next-less&repository-name=with-next-less)
diff --git a/examples/with-next-page-transitions/README.md b/examples/with-next-page-transitions/README.md
index 2556c5264533c..8637a3fc0fb61 100644
--- a/examples/with-next-page-transitions/README.md
+++ b/examples/with-next-page-transitions/README.md
@@ -6,7 +6,7 @@ This example includes two pages with links between them. The "About" page demons
## Deploy your own
-Deploy the example using [Vercel](https://vercel.com):
+Deploy the example using [Vercel](https://vercel.com?utm_source=github&utm_medium=readme&utm_campaign=next-example):
[](https://vercel.com/new/git/external?repository-url=https://github.com/vercel/next.js/tree/canary/examples/with-next-page-transitions&project-name=with-next-page-transitions&repository-name=with-next-page-transitions)
diff --git a/examples/with-next-sass/README.md b/examples/with-next-sass/README.md
index 168a4cd3d2adc..d28ee63b95b75 100644
--- a/examples/with-next-sass/README.md
+++ b/examples/with-next-sass/README.md
@@ -4,7 +4,7 @@ This example demonstrates how to use Next.js' built-in Global Sass/Scss imports
## Deploy your own
-Deploy the example using [Vercel](https://vercel.com):
+Deploy the example using [Vercel](https://vercel.com?utm_source=github&utm_medium=readme&utm_campaign=next-example):
[](https://vercel.com/new/git/external?repository-url=https://github.com/vercel/next.js/tree/canary/examples/with-next-sass&project-name=with-next-sass&repository-name=with-next-sass)
diff --git a/examples/with-next-seo/README.md b/examples/with-next-seo/README.md
index ca2a29906264e..ac45eb91f4f37 100644
--- a/examples/with-next-seo/README.md
+++ b/examples/with-next-seo/README.md
@@ -4,7 +4,7 @@ This example shows how you integrate [next-seo](https://github.com/garmeeh/next-
## Deploy your own
-Deploy the example using [Vercel](https://vercel.com):
+Deploy the example using [Vercel](https://vercel.com?utm_source=github&utm_medium=readme&utm_campaign=next-example):
[](https://vercel.com/new/git/external?repository-url=https://github.com/vercel/next.js/tree/canary/examples/with-next-seo&project-name=with-next-seo&repository-name=with-next-seo)
diff --git a/examples/with-next-sitemap/README.md b/examples/with-next-sitemap/README.md
index de526276ec060..89b3f18cd4978 100644
--- a/examples/with-next-sitemap/README.md
+++ b/examples/with-next-sitemap/README.md
@@ -6,7 +6,7 @@ This example uses [`next-sitemap`](https://github.com/iamvishnusankar/next-sitem
## Deploy your own
-Deploy the example using [Vercel](https://vercel.com):
+Deploy the example using [Vercel](https://vercel.com?utm_source=github&utm_medium=readme&utm_campaign=next-example):
[](https://vercel.com/new/git/external?repository-url=https://github.com/vercel/next.js/tree/canary/examples/with-next-sitemap&project-name=with-next-sitemap&repository-name=with-next-sitemap)
diff --git a/examples/with-next-translate/README.md b/examples/with-next-translate/README.md
index 91501881a55f0..5d8a46fbcf5fa 100644
--- a/examples/with-next-translate/README.md
+++ b/examples/with-next-translate/README.md
@@ -4,7 +4,7 @@ This is an example of using [next-translate](https://github.com/vinissimus/next-
## Deploy your own
-Deploy the example using [Vercel](https://vercel.com):
+Deploy the example using [Vercel](https://vercel.com?utm_source=github&utm_medium=readme&utm_campaign=next-example):
[](https://vercel.com/new/git/external?repository-url=https://github.com/vercel/next.js/tree/canary/examples/with-next-translate&project-name=with-next-translate&repository-name=with-next-translate)
diff --git a/examples/with-orbit-components/README.md b/examples/with-orbit-components/README.md
index 42a40d5b40fee..4665b477b2689 100644
--- a/examples/with-orbit-components/README.md
+++ b/examples/with-orbit-components/README.md
@@ -8,7 +8,7 @@ This fork comes from [styled-components-example](https://github.com/vercel/next.
## Deploy your own
-Deploy the example using [Vercel](https://vercel.com):
+Deploy the example using [Vercel](https://vercel.com?utm_source=github&utm_medium=readme&utm_campaign=next-example):
[](https://vercel.com/new/git/external?repository-url=https://github.com/vercel/next.js/tree/canary/examples/with-orbit-components&project-name=with-orbit-components&repository-name=with-orbit-components)
diff --git a/examples/with-overmind/README.md b/examples/with-overmind/README.md
index 83f735aab7f8a..0c499897ed1b8 100644
--- a/examples/with-overmind/README.md
+++ b/examples/with-overmind/README.md
@@ -4,7 +4,7 @@ This example uses [overmind](https://overmindjs.org/?view=react&typescript=false
## Deploy your own
-Deploy the example using [Vercel](https://vercel.com):
+Deploy the example using [Vercel](https://vercel.com?utm_source=github&utm_medium=readme&utm_campaign=next-example):
[](https://vercel.com/new/git/external?repository-url=https://github.com/vercel/next.js/tree/canary/examples/with-overmind&project-name=with-overmind&repository-name=with-overmind)
diff --git a/examples/with-passport-and-next-connect/README.md b/examples/with-passport-and-next-connect/README.md
index e44585ddf4d37..69d01f136c6e4 100644
--- a/examples/with-passport-and-next-connect/README.md
+++ b/examples/with-passport-and-next-connect/README.md
@@ -8,7 +8,7 @@ For demo purpose, the users database is stored in the cookie session. You need t
## Deploy your own
-Deploy the example using [Vercel](https://vercel.com):
+Deploy the example using [Vercel](https://vercel.com?utm_source=github&utm_medium=readme&utm_campaign=next-example):
[](https://vercel.com/new/git/external?repository-url=https://github.com/vercel/next.js/tree/canary/examples/with-passport-and-next-connect&project-name=with-passport-and-next-connect&repository-name=with-passport-and-next-connect)
diff --git a/examples/with-passport/README.md b/examples/with-passport/README.md
index fb40a130cbd39..5f00342abc712 100644
--- a/examples/with-passport/README.md
+++ b/examples/with-passport/README.md
@@ -10,7 +10,7 @@ The login cookie is httpOnly, meaning it can only be accessed by the API, and it
## Deploy your own
-Deploy the example using [Vercel](https://vercel.com):
+Deploy the example using [Vercel](https://vercel.com?utm_source=github&utm_medium=readme&utm_campaign=next-example):
[](https://vercel.com/new/git/external?repository-url=https://github.com/vercel/next.js/tree/canary/examples/with-passport&project-name=with-passport&repository-name=with-passport)
diff --git a/examples/with-paste-typescript/README.md b/examples/with-paste-typescript/README.md
index 0e60aff397794..6e920e9a12af1 100644
--- a/examples/with-paste-typescript/README.md
+++ b/examples/with-paste-typescript/README.md
@@ -8,7 +8,7 @@ Next.js and Paste have built-in TypeScript declarations, so you'll get autocompl
## Deploy your own
-Deploy the example using [Vercel](https://vercel.com):
+Deploy the example using [Vercel](https://vercel.com?utm_source=github&utm_medium=readme&utm_campaign=next-example):
[](https://vercel.com/new/git/external?repository-url=https://github.com/vercel/next.js/tree/canary/examples/with-paste-typescript&project-name=with-paste-typescript&repository-name=with-paste-typescript)
diff --git a/examples/with-patternfly/README.md b/examples/with-patternfly/README.md
index 5eb202cc764d2..71e8116f9fcd6 100644
--- a/examples/with-patternfly/README.md
+++ b/examples/with-patternfly/README.md
@@ -4,7 +4,7 @@ This example shows how to use Next.js along with [Patterfly](https://www.pattern
## Deploy your own
-Deploy the example using [Vercel](https://vercel.com):
+Deploy the example using [Vercel](https://vercel.com?utm_source=github&utm_medium=readme&utm_campaign=next-example):
[](https://vercel.com/new/git/external?repository-url=https://github.com/vercel/next.js/tree/canary/examples/with-patternfly&project-name=with-patternfly&repository-name=with-patternfly)
diff --git a/examples/with-polyfills/README.md b/examples/with-polyfills/README.md
index eb5df31a8799c..24db2515b9cf9 100644
--- a/examples/with-polyfills/README.md
+++ b/examples/with-polyfills/README.md
@@ -8,7 +8,7 @@ In this case, you should add a top-level import for the specific polyfill you ne
## Deploy your own
-Deploy the example using [Vercel](https://vercel.com):
+Deploy the example using [Vercel](https://vercel.com?utm_source=github&utm_medium=readme&utm_campaign=next-example):
[](https://vercel.com/new/git/external?repository-url=https://github.com/vercel/next.js/tree/canary/examples/with-polyfills&project-name=with-polyfills&repository-name=with-polyfills)
diff --git a/examples/with-portals-ssr/README.md b/examples/with-portals-ssr/README.md
index 2778ad37cce15..8a81d45b4019c 100644
--- a/examples/with-portals-ssr/README.md
+++ b/examples/with-portals-ssr/README.md
@@ -4,7 +4,7 @@ An example of Server Side Rendered React [Portals](https://reactjs.org/docs/port
## Deploy your own
-Deploy the example using [Vercel](https://vercel.com):
+Deploy the example using [Vercel](https://vercel.com?utm_source=github&utm_medium=readme&utm_campaign=next-example):
[](https://vercel.com/new/git/external?repository-url=https://github.com/vercel/next.js/tree/canary/examples/with-portals-ssr&project-name=with-portals-ssr&repository-name=with-portals-ssr)
diff --git a/examples/with-portals/README.md b/examples/with-portals/README.md
index 9d04bad5fa37c..f77dc924fe77d 100644
--- a/examples/with-portals/README.md
+++ b/examples/with-portals/README.md
@@ -4,7 +4,7 @@ This example show how to use the React [Portals](https://reactjs.org/docs/portal
## Deploy your own
-Deploy the example using [Vercel](https://vercel.com):
+Deploy the example using [Vercel](https://vercel.com?utm_source=github&utm_medium=readme&utm_campaign=next-example):
[](https://vercel.com/new/git/external?repository-url=https://github.com/vercel/next.js/tree/canary/examples/with-portals&project-name=with-portals&repository-name=with-portals)
diff --git a/examples/with-prefetching/README.md b/examples/with-prefetching/README.md
index f6c6a2cd37141..5a53f5879c2f6 100644
--- a/examples/with-prefetching/README.md
+++ b/examples/with-prefetching/README.md
@@ -8,7 +8,7 @@ This example features an app with four simple pages:
## Deploy your own
-Deploy the example using [Vercel](https://vercel.com):
+Deploy the example using [Vercel](https://vercel.com?utm_source=github&utm_medium=readme&utm_campaign=next-example):
[](https://vercel.com/new/git/external?repository-url=https://github.com/vercel/next.js/tree/canary/examples/with-prefetching&project-name=with-prefetching&repository-name=with-prefetching)
diff --git a/examples/with-quill-js/README.md b/examples/with-quill-js/README.md
index d6947527d8e62..cbacba13a1e49 100644
--- a/examples/with-quill-js/README.md
+++ b/examples/with-quill-js/README.md
@@ -6,7 +6,7 @@ Quill does not suppport SSR, so it's only loaded and rendered in the browser.
## Deploy your own
-Deploy the example using [Vercel](https://vercel.com):
+Deploy the example using [Vercel](https://vercel.com?utm_source=github&utm_medium=readme&utm_campaign=next-example):
[](https://vercel.com/new/git/external?repository-url=https://github.com/vercel/next.js/tree/canary/examples/with-quill-js&project-name=with-quill-js&repository-name=with-quill-js)
diff --git a/examples/with-rbx-bulma-pro/README.md b/examples/with-rbx-bulma-pro/README.md
index 7f5b6eab4e658..c363859e156ce 100644
--- a/examples/with-rbx-bulma-pro/README.md
+++ b/examples/with-rbx-bulma-pro/README.md
@@ -4,7 +4,7 @@ This example shows how to use Next.js along with [rbx](https://github.com/dfee/r
## Deploy your own
-Deploy the example using [Vercel](https://vercel.com):
+Deploy the example using [Vercel](https://vercel.com?utm_source=github&utm_medium=readme&utm_campaign=next-example):
[](https://vercel.com/new/git/external?repository-url=https://github.com/vercel/next.js/tree/canary/examples/with-rbx-bulma-pro&project-name=with-rbx-bulma-pro&repository-name=with-rbx-bulma-pro)
diff --git a/examples/with-react-bootstrap/README.md b/examples/with-react-bootstrap/README.md
index d398a8aba2094..1f0106cc1e509 100644
--- a/examples/with-react-bootstrap/README.md
+++ b/examples/with-react-bootstrap/README.md
@@ -4,7 +4,7 @@ This example shows how to use Next.js along with [react-bootstrap](https://react
## Deploy your own
-Deploy the example using [Vercel](https://vercel.com):
+Deploy the example using [Vercel](https://vercel.com?utm_source=github&utm_medium=readme&utm_campaign=next-example):
[](https://vercel.com/new/git/external?repository-url=https://github.com/vercel/next.js/tree/canary/examples/with-react-bootstrap&project-name=with-react-bootstrap&repository-name=with-react-bootstrap)
diff --git a/examples/with-react-ga/README.md b/examples/with-react-ga/README.md
index 91f735eb5ba0a..11c37b302f0a1 100644
--- a/examples/with-react-ga/README.md
+++ b/examples/with-react-ga/README.md
@@ -5,7 +5,7 @@ component with NextJs. Modify `Tracking ID` in `utils/analytics.js` file for tes
## Deploy your own
-Deploy the example using [Vercel](https://vercel.com):
+Deploy the example using [Vercel](https://vercel.com?utm_source=github&utm_medium=readme&utm_campaign=next-example):
[](https://vercel.com/new/git/external?repository-url=https://github.com/vercel/next.js/tree/canary/examples/with-react-ga&project-name=with-react-ga&repository-name=with-react-ga)
diff --git a/examples/with-react-helmet/README.md b/examples/with-react-helmet/README.md
index a4b9a09ca732f..d18fca27cf42b 100644
--- a/examples/with-react-helmet/README.md
+++ b/examples/with-react-helmet/README.md
@@ -7,7 +7,7 @@ The rest is all up to you.
## Deploy your own
-Deploy the example using [Vercel](https://vercel.com):
+Deploy the example using [Vercel](https://vercel.com?utm_source=github&utm_medium=readme&utm_campaign=next-example):
[](https://vercel.com/new/git/external?repository-url=https://github.com/vercel/next.js/tree/canary/examples/with-react-helmet&project-name=with-react-helmet&repository-name=with-react-helmet)
diff --git a/examples/with-react-jss/README.md b/examples/with-react-jss/README.md
index 78efdda015230..c071f5e4d93a2 100644
--- a/examples/with-react-jss/README.md
+++ b/examples/with-react-jss/README.md
@@ -6,7 +6,7 @@ The critical styles will be injected into the head when server rendered.
## Deploy your own
-Deploy the example using [Vercel](https://vercel.com):
+Deploy the example using [Vercel](https://vercel.com?utm_source=github&utm_medium=readme&utm_campaign=next-example):
[](https://vercel.com/new/git/external?repository-url=https://github.com/vercel/next.js/tree/canary/examples/with-react-jss&project-name=with-react-jss&repository-name=with-react-jss)
diff --git a/examples/with-react-multi-carousel/README.md b/examples/with-react-multi-carousel/README.md
index 14469508a99e8..9a54079c42d9f 100644
--- a/examples/with-react-multi-carousel/README.md
+++ b/examples/with-react-multi-carousel/README.md
@@ -4,7 +4,7 @@
## Deploy your own
-Deploy the example using [Vercel](https://vercel.com):
+Deploy the example using [Vercel](https://vercel.com?utm_source=github&utm_medium=readme&utm_campaign=next-example):
[](https://vercel.com/new/git/external?repository-url=https://github.com/vercel/next.js/tree/canary/examples/with-react-multi-carousel&project-name=with-react-multi-carousel&repository-name=with-react-multi-carousel)
diff --git a/examples/with-react-native-web/README.md b/examples/with-react-native-web/README.md
index e89378e2870bc..525ba1a7c4004 100644
--- a/examples/with-react-native-web/README.md
+++ b/examples/with-react-native-web/README.md
@@ -8,7 +8,7 @@ This example features how to use [react-native-web](https://github.com/necolas/r
## Deploy your own
-Deploy the example using [Vercel](https://vercel.com):
+Deploy the example using [Vercel](https://vercel.com?utm_source=github&utm_medium=readme&utm_campaign=next-example):
[](https://vercel.com/new/git/external?repository-url=https://github.com/vercel/next.js/tree/canary/examples/with-react-native-web&project-name=with-react-native-web&repository-name=with-react-native-web)
diff --git a/examples/with-react-relay-network-modern/README.md b/examples/with-react-relay-network-modern/README.md
index e05001215ad1f..7684104bb8e8b 100644
--- a/examples/with-react-relay-network-modern/README.md
+++ b/examples/with-react-relay-network-modern/README.md
@@ -6,7 +6,7 @@ This example relies on [Prisma + Nexus](https://github.com/prisma-labs/nextjs-gr
## Deploy your own
-Deploy the example using [Vercel](https://vercel.com):
+Deploy the example using [Vercel](https://vercel.com?utm_source=github&utm_medium=readme&utm_campaign=next-example):
[](https://vercel.com/new/git/external?repository-url=https://github.com/vercel/next.js/tree/canary/examples/with-react-relay-network-modern&project-name=with-react-relay-network-modern&repository-name=with-react-relay-network-modern)
diff --git a/examples/with-react-toolbox/README.md b/examples/with-react-toolbox/README.md
index b1ad7f9ea5e89..184b7d62adad9 100644
--- a/examples/with-react-toolbox/README.md
+++ b/examples/with-react-toolbox/README.md
@@ -6,7 +6,7 @@ For actual use, you probably also want to add Roboto Font, and Material Design I
## Deploy your own
-Deploy the example using [Vercel](https://vercel.com):
+Deploy the example using [Vercel](https://vercel.com?utm_source=github&utm_medium=readme&utm_campaign=next-example):
[](https://vercel.com/new/git/external?repository-url=https://github.com/vercel/next.js/tree/canary/examples/with-react-toolbox&project-name=with-react-toolbox&repository-name=with-react-toolbox)
diff --git a/examples/with-react-with-styles/README.md b/examples/with-react-with-styles/README.md
index 8f38d0d2cdb8b..4b60c60c6f89a 100644
--- a/examples/with-react-with-styles/README.md
+++ b/examples/with-react-with-styles/README.md
@@ -10,7 +10,7 @@ We are using `pages/_index.js` from this example [with-aphrodite](https://github
## Deploy your own
-Deploy the example using [Vercel](https://vercel.com):
+Deploy the example using [Vercel](https://vercel.com?utm_source=github&utm_medium=readme&utm_campaign=next-example):
[](https://vercel.com/new/git/external?repository-url=https://github.com/vercel/next.js/tree/canary/examples/with-react-with-styles&project-name=with-react-with-styles&repository-name=with-react-with-styles)
diff --git a/examples/with-reactstrap/README.md b/examples/with-reactstrap/README.md
index 103ed59d3b1e6..ef3a42ec9c0a0 100644
--- a/examples/with-reactstrap/README.md
+++ b/examples/with-reactstrap/README.md
@@ -4,7 +4,7 @@ This example shows how to use Next.js with [reactstrap](https://reactstrap.githu
## Deploy your own
-Deploy the example using [Vercel](https://vercel.com):
+Deploy the example using [Vercel](https://vercel.com?utm_source=github&utm_medium=readme&utm_campaign=next-example):
[](https://vercel.com/new/git/external?repository-url=https://github.com/vercel/next.js/tree/canary/examples/with-reactstrap&project-name=with-reactstrap&repository-name=with-reactstrap)
diff --git a/examples/with-realm-web/README.md b/examples/with-realm-web/README.md
index 0716e39b81c0d..5f48249cde46a 100644
--- a/examples/with-realm-web/README.md
+++ b/examples/with-realm-web/README.md
@@ -6,7 +6,7 @@ This example relies on [MongoDB Realm](https://www.mongodb.com/realm) for its Gr
## Deploy your own
-Deploy the example using [Vercel](https://vercel.com):
+Deploy the example using [Vercel](https://vercel.com?utm_source=github&utm_medium=readme&utm_campaign=next-example):
[](https://vercel.com/new/git/external?repository-url=https://github.com/vercel/next.js/tree/canary/examples/with-realm-web&project-name=with-realm-web&repository-name=with-realm-web)
diff --git a/examples/with-reason-relay/README.md b/examples/with-reason-relay/README.md
index 6386c862fcf58..e72a4a374bfcc 100644
--- a/examples/with-reason-relay/README.md
+++ b/examples/with-reason-relay/README.md
@@ -6,7 +6,7 @@ This example relies on [Prisma + Nexus](https://github.com/prisma-labs/nextjs-gr
## Deploy your own
-Deploy the example using [Vercel](https://vercel.com):
+Deploy the example using [Vercel](https://vercel.com?utm_source=github&utm_medium=readme&utm_campaign=next-example):
[](https://vercel.com/new/git/external?repository-url=https://github.com/vercel/next.js/tree/canary/examples/with-reason-relay&project-name=with-reason-relay&repository-name=with-reason-relay)
diff --git a/examples/with-reasonml-todo/README.md b/examples/with-reasonml-todo/README.md
index e75c655c765ed..a7aa61234949e 100644
--- a/examples/with-reasonml-todo/README.md
+++ b/examples/with-reasonml-todo/README.md
@@ -16,7 +16,7 @@ This example features:
## Deploy your own
-Deploy the example using [Vercel](https://vercel.com):
+Deploy the example using [Vercel](https://vercel.com?utm_source=github&utm_medium=readme&utm_campaign=next-example):
[](https://vercel.com/new/git/external?repository-url=https://github.com/vercel/next.js/tree/canary/examples/with-reasonml-todo&project-name=with-reasonml-todo&repository-name=with-reasonml-todo)
diff --git a/examples/with-reasonml/README.md b/examples/with-reasonml/README.md
index e015b8761978b..bab95e3447e6e 100644
--- a/examples/with-reasonml/README.md
+++ b/examples/with-reasonml/README.md
@@ -10,7 +10,7 @@ This example features:
## Deploy your own
-Deploy the example using [Vercel](https://vercel.com):
+Deploy the example using [Vercel](https://vercel.com?utm_source=github&utm_medium=readme&utm_campaign=next-example):
[](https://vercel.com/new/git/external?repository-url=https://github.com/vercel/next.js/tree/canary/examples/with-reasonml&project-name=with-reasonml&repository-name=with-reasonml)
diff --git a/examples/with-rebass/README.md b/examples/with-rebass/README.md
index ff6365639d648..28aa0023cbefa 100644
--- a/examples/with-rebass/README.md
+++ b/examples/with-rebass/README.md
@@ -6,7 +6,7 @@ This example features how you use [Rebass](https://jxnblk.com/rebass/) functiona
## Deploy your own
-Deploy the example using [Vercel](https://vercel.com):
+Deploy the example using [Vercel](https://vercel.com?utm_source=github&utm_medium=readme&utm_campaign=next-example):
[](https://vercel.com/new/git/external?repository-url=https://github.com/vercel/next.js/tree/canary/examples/with-rebass&project-name=with-rebass&repository-name=with-rebass)
diff --git a/examples/with-recoil/README.md b/examples/with-recoil/README.md
index f1373b45f203b..b28b85b9eb587 100644
--- a/examples/with-recoil/README.md
+++ b/examples/with-recoil/README.md
@@ -6,7 +6,7 @@ This example shows how to integrate Recoil in Next.js.
## Deploy your own
-Deploy the example using [Vercel](https://vercel.com):
+Deploy the example using [Vercel](https://vercel.com?utm_source=github&utm_medium=readme&utm_campaign=next-example):
[](https://vercel.com/new/git/external?repository-url=https://github.com/vercel/next.js/tree/canary/examples/with-recoil&project-name=with-recoil&repository-name=with-recoil)
diff --git a/examples/with-redux-code-splitting/README.md b/examples/with-redux-code-splitting/README.md
index b4ffd0c26a558..c9cf1e10bf22e 100644
--- a/examples/with-redux-code-splitting/README.md
+++ b/examples/with-redux-code-splitting/README.md
@@ -6,7 +6,7 @@ This example utilizes [fast-redux](https://github.com/dogada/fast-redux) to spli
## Deploy your own
-Deploy the example using [Vercel](https://vercel.com):
+Deploy the example using [Vercel](https://vercel.com?utm_source=github&utm_medium=readme&utm_campaign=next-example):
[](https://vercel.com/new/git/external?repository-url=https://github.com/vercel/next.js/tree/canary/examples/with-redux-code-splitting&project-name=with-redux-code-splitting&repository-name=with-redux-code-splitting)
diff --git a/examples/with-redux-observable/README.md b/examples/with-redux-observable/README.md
index 541890c993961..2518db031b8dd 100644
--- a/examples/with-redux-observable/README.md
+++ b/examples/with-redux-observable/README.md
@@ -10,7 +10,7 @@ Example also uses `redux-logger` to log every action.
## Deploy your own
-Deploy the example using [Vercel](https://vercel.com):
+Deploy the example using [Vercel](https://vercel.com?utm_source=github&utm_medium=readme&utm_campaign=next-example):
[](https://vercel.com/new/git/external?repository-url=https://github.com/vercel/next.js/tree/canary/examples/with-redux-observable&project-name=with-redux-observable&repository-name=with-redux-observable)
diff --git a/examples/with-redux-persist/README.md b/examples/with-redux-persist/README.md
index 36ecd4c891bab..adeca8876e98b 100644
--- a/examples/with-redux-persist/README.md
+++ b/examples/with-redux-persist/README.md
@@ -6,7 +6,7 @@ With the advantage of having a global state for your app using `redux`. You'll a
## Deploy your own
-Deploy the example using [Vercel](https://vercel.com):
+Deploy the example using [Vercel](https://vercel.com?utm_source=github&utm_medium=readme&utm_campaign=next-example):
[](https://vercel.com/new/git/external?repository-url=https://github.com/vercel/next.js/tree/canary/examples/with-redux-persist&project-name=with-redux-persist&repository-name=with-redux-persist)
diff --git a/examples/with-redux-saga/README.md b/examples/with-redux-saga/README.md
index 971c79eaa0a03..b72b990da8196 100644
--- a/examples/with-redux-saga/README.md
+++ b/examples/with-redux-saga/README.md
@@ -6,7 +6,7 @@ Usually splitting your app state into `pages` feels natural, but sometimes you'l
## Deploy your own
-Deploy the example using [Vercel](https://vercel.com):
+Deploy the example using [Vercel](https://vercel.com?utm_source=github&utm_medium=readme&utm_campaign=next-example):
[](https://vercel.com/new/git/external?repository-url=https://github.com/vercel/next.js/tree/canary/examples/with-redux-saga&project-name=with-redux-saga&repository-name=with-redux-saga)
diff --git a/examples/with-redux-thunk/README.md b/examples/with-redux-thunk/README.md
index 681bb4e8ff668..176eb86d1a598 100644
--- a/examples/with-redux-thunk/README.md
+++ b/examples/with-redux-thunk/README.md
@@ -6,7 +6,7 @@ Usually splitting your app state into `pages` feels natural but sometimes you'll
## Deploy your own
-Deploy the example using [Vercel](https://vercel.com):
+Deploy the example using [Vercel](https://vercel.com?utm_source=github&utm_medium=readme&utm_campaign=next-example):
[](https://vercel.com/new/git/external?repository-url=https://github.com/vercel/next.js/tree/canary/examples/with-redux-thunk&project-name=with-redux-thunk&repository-name=with-redux-thunk)
diff --git a/examples/with-redux-toolkit/README.md b/examples/with-redux-toolkit/README.md
index a8f3887264a07..c0a6a3b9f3a0f 100644
--- a/examples/with-redux-toolkit/README.md
+++ b/examples/with-redux-toolkit/README.md
@@ -6,7 +6,7 @@ The **Redux Toolkit** is intended to be the standard way to write Redux logic (c
## Deploy your own
-Deploy the example using [Vercel](https://vercel.com):
+Deploy the example using [Vercel](https://vercel.com?utm_source=github&utm_medium=readme&utm_campaign=next-example):
[](https://vercel.com/new/git/external?repository-url=https://github.com/vercel/next.js/tree/canary/examples/with-redux-toolkit&project-name=with-redux-toolkit&repository-name=with-redux-toolkit)
diff --git a/examples/with-redux-wrapper/README.md b/examples/with-redux-wrapper/README.md
index bad33656b5a79..d2d2e91735b64 100644
--- a/examples/with-redux-wrapper/README.md
+++ b/examples/with-redux-wrapper/README.md
@@ -4,7 +4,7 @@ Usually splitting your app state into `pages` feels natural but sometimes you'll
## Deploy your own
-Deploy the example using [Vercel](https://vercel.com):
+Deploy the example using [Vercel](https://vercel.com?utm_source=github&utm_medium=readme&utm_campaign=next-example):
[](https://vercel.com/new/git/external?repository-url=https://github.com/vercel/next.js/tree/canary/examples/with-redux-wrapper&project-name=with-redux-wrapper&repository-name=with-redux-wrapper)
diff --git a/examples/with-redux/README.md b/examples/with-redux/README.md
index 21d3dc2791047..36106fb648a59 100644
--- a/examples/with-redux/README.md
+++ b/examples/with-redux/README.md
@@ -16,7 +16,7 @@ On the server side every request initializes a new store, because otherwise diff
## Deploy your own
-Deploy the example using [Vercel](https://vercel.com):
+Deploy the example using [Vercel](https://vercel.com?utm_source=github&utm_medium=readme&utm_campaign=next-example):
[](https://vercel.com/new/git/external?repository-url=https://github.com/vercel/next.js/tree/canary/examples/with-redux&project-name=with-redux&repository-name=with-redux)
diff --git a/examples/with-reflux/README.md b/examples/with-reflux/README.md
index 5287f05d304ab..ac077bdd010ce 100644
--- a/examples/with-reflux/README.md
+++ b/examples/with-reflux/README.md
@@ -4,7 +4,7 @@ Use [reflux](https://github.com/reflux/refluxjs) to manage an application store
## Deploy your own
-Deploy the example using [Vercel](https://vercel.com):
+Deploy the example using [Vercel](https://vercel.com?utm_source=github&utm_medium=readme&utm_campaign=next-example):
[](https://vercel.com/new/git/external?repository-url=https://github.com/vercel/next.js/tree/canary/examples/with-reflux&project-name=with-reflux&repository-name=with-reflux)
diff --git a/examples/with-relay-modern/README.md b/examples/with-relay-modern/README.md
index 51c98422264ba..4f95a7bbe5aed 100644
--- a/examples/with-relay-modern/README.md
+++ b/examples/with-relay-modern/README.md
@@ -6,7 +6,7 @@ This example relies on [Prisma + Nexus](https://github.com/prisma-labs/nextjs-gr
## Deploy your own
-Deploy the example using [Vercel](https://vercel.com):
+Deploy the example using [Vercel](https://vercel.com?utm_source=github&utm_medium=readme&utm_campaign=next-example):
[](https://vercel.com/new/git/external?repository-url=https://github.com/vercel/next.js/tree/canary/examples/with-relay-modern&project-name=with-relay-modern&repository-name=with-relay-modern)
diff --git a/examples/with-rematch/README.md b/examples/with-rematch/README.md
index 0492c44b0dc88..9e526c1e2a5c7 100644
--- a/examples/with-rematch/README.md
+++ b/examples/with-rematch/README.md
@@ -6,7 +6,7 @@ Since rematch is utility which uses redux under the hood, some elements like `st
## Deploy your own
-Deploy the example using [Vercel](https://vercel.com):
+Deploy the example using [Vercel](https://vercel.com?utm_source=github&utm_medium=readme&utm_campaign=next-example):
[](https://vercel.com/new/git/external?repository-url=https://github.com/vercel/next.js/tree/canary/examples/with-rematch&project-name=with-rematch&repository-name=with-rematch)
diff --git a/examples/with-route-as-modal/README.md b/examples/with-route-as-modal/README.md
index 6ac5eea9ce9e4..b228d78adb7ee 100644
--- a/examples/with-route-as-modal/README.md
+++ b/examples/with-route-as-modal/README.md
@@ -6,7 +6,7 @@ This example shows how to conditionally display a modal based on a route.
## Deploy your own
-Deploy the example using [Vercel](https://vercel.com):
+Deploy the example using [Vercel](https://vercel.com?utm_source=github&utm_medium=readme&utm_campaign=next-example):
[](https://vercel.com/new/git/external?repository-url=https://github.com/vercel/next.js/tree/canary/examples/with-route-as-modal&project-name=with-route-as-modal&repository-name=with-route-as-modal)
diff --git a/examples/with-segment-analytics/README.md b/examples/with-segment-analytics/README.md
index c84c03d68281e..e731d4f003e1f 100644
--- a/examples/with-segment-analytics/README.md
+++ b/examples/with-segment-analytics/README.md
@@ -4,7 +4,7 @@ This example shows how to use Next.js along with [Segment Analytics](https://seg
## Deploy your own
-Deploy the example using [Vercel](https://vercel.com):
+Deploy the example using [Vercel](https://vercel.com?utm_source=github&utm_medium=readme&utm_campaign=next-example):
[](https://vercel.com/new/git/external?repository-url=https://github.com/vercel/next.js/tree/canary/examples/with-segment-analytics&project-name=with-segment-analytics&repository-name=with-segment-analytics)
diff --git a/examples/with-semantic-ui/README.md b/examples/with-semantic-ui/README.md
index 9e5b5d31b7e00..23edfa6b1e50a 100644
--- a/examples/with-semantic-ui/README.md
+++ b/examples/with-semantic-ui/README.md
@@ -4,7 +4,7 @@ This example shows how to use Next.js along with [Semantic UI React](https://rea
## Deploy your own
-Deploy the example using [Vercel](https://vercel.com):
+Deploy the example using [Vercel](https://vercel.com?utm_source=github&utm_medium=readme&utm_campaign=next-example):
[](https://vercel.com/new/git/external?repository-url=https://github.com/vercel/next.js/tree/canary/examples/with-semantic-ui&project-name=with-semantic-ui&repository-name=with-semantic-ui)
diff --git a/examples/with-shallow-routing/README.md b/examples/with-shallow-routing/README.md
index e1c6028ece89c..4387a6ac64bed 100644
--- a/examples/with-shallow-routing/README.md
+++ b/examples/with-shallow-routing/README.md
@@ -6,7 +6,7 @@ We do this by passing the `shallow: true` option to `Router.push` or `Router.rep
## Deploy your own
-Deploy the example using [Vercel](https://vercel.com):
+Deploy the example using [Vercel](https://vercel.com?utm_source=github&utm_medium=readme&utm_campaign=next-example):
[](https://vercel.com/new/git/external?repository-url=https://github.com/vercel/next.js/tree/canary/examples/with-shallow-routing&project-name=with-shallow-routing&repository-name=with-shallow-routing)
diff --git a/examples/with-sitemap/README.md b/examples/with-sitemap/README.md
index c70b49bbc90b9..3e57d6207f6a4 100644
--- a/examples/with-sitemap/README.md
+++ b/examples/with-sitemap/README.md
@@ -4,7 +4,7 @@ This example shows how to generate a `sitemap.xml` file based on the pages in yo
## Deploy your own
-Deploy the example using [Vercel](https://vercel.com):
+Deploy the example using [Vercel](https://vercel.com?utm_source=github&utm_medium=readme&utm_campaign=next-example):
[](https://vercel.com/new/git/external?repository-url=https://github.com/vercel/next.js/tree/canary/examples/with-sitemap&project-name=with-sitemap&repository-name=with-sitemap)
diff --git a/examples/with-slate/README.md b/examples/with-slate/README.md
index af0782ed39972..c0eca0a04e53d 100644
--- a/examples/with-slate/README.md
+++ b/examples/with-slate/README.md
@@ -4,7 +4,7 @@ This example shows how to use Next.js along with [Slate.js](https://www.slatejs.
## Deploy your own
-Deploy the example using [Vercel](https://vercel.com):
+Deploy the example using [Vercel](https://vercel.com?utm_source=github&utm_medium=readme&utm_campaign=next-example):
[](https://vercel.com/new/git/external?repository-url=https://github.com/vercel/next.js/tree/canary/examples/with-slate&project-name=with-slate&repository-name=with-slate)
diff --git a/examples/with-stencil/README.md b/examples/with-stencil/README.md
index ed5276f561ef8..43328e35324f9 100644
--- a/examples/with-stencil/README.md
+++ b/examples/with-stencil/README.md
@@ -2,7 +2,7 @@
## Deploy your own
-Deploy the example using [Vercel](https://vercel.com):
+Deploy the example using [Vercel](https://vercel.com?utm_source=github&utm_medium=readme&utm_campaign=next-example):
[](https://vercel.com/new/git/external?repository-url=https://github.com/vercel/next.js/tree/canary/examples/with-stencil&project-name=with-stencil&repository-name=with-stencil)
diff --git a/examples/with-stitches/README.md b/examples/with-stitches/README.md
index 2e58569ee96a7..c9b72087a8bd4 100644
--- a/examples/with-stitches/README.md
+++ b/examples/with-stitches/README.md
@@ -4,7 +4,7 @@ This example shows how to use the [Stitches CSS-in-JS Library](https://github.co
## Deploy your own
-Deploy the example using [Vercel](https://vercel.com):
+Deploy the example using [Vercel](https://vercel.com?utm_source=github&utm_medium=readme&utm_campaign=next-example):
[](https://vercel.com/new/git/external?repository-url=https://github.com/vercel/next.js/tree/canary/examples/with-stitches&project-name=with-stitches&repository-name=with-stitches)
diff --git a/examples/with-storybook-styled-jsx-scss/README.md b/examples/with-storybook-styled-jsx-scss/README.md
index aa0d7d69dbef0..46432231b4cac 100644
--- a/examples/with-storybook-styled-jsx-scss/README.md
+++ b/examples/with-storybook-styled-jsx-scss/README.md
@@ -4,7 +4,7 @@ This example shows Styled-jsx (with SCSS) working for components written in Type
## Deploy your own
-Deploy the example using [Vercel](https://vercel.com):
+Deploy the example using [Vercel](https://vercel.com?utm_source=github&utm_medium=readme&utm_campaign=next-example):
[](https://vercel.com/new/git/external?repository-url=https://github.com/vercel/next.js/tree/canary/examples/with-storybook-styled-jsx-scss&project-name=with-storybook-styled-jsx-scss&repository-name=with-storybook-styled-jsx-scss)
diff --git a/examples/with-storybook/README.md b/examples/with-storybook/README.md
index 2c92d748cb047..7f839e294b4c1 100644
--- a/examples/with-storybook/README.md
+++ b/examples/with-storybook/README.md
@@ -8,7 +8,7 @@ As of v6.0, Storybook has built-in TypeScript support, so no configuration is ne
## Deploy your own
-Deploy the example using [Vercel](https://vercel.com):
+Deploy the example using [Vercel](https://vercel.com?utm_source=github&utm_medium=readme&utm_campaign=next-example):
[](https://vercel.com/new/git/external?repository-url=https://github.com/vercel/next.js/tree/canary/examples/with-storybook&project-name=with-storybook&repository-name=with-storybook)
diff --git a/examples/with-strict-csp/README.md b/examples/with-strict-csp/README.md
index 9ecbaa93f2202..9b2ee4363a4bb 100644
--- a/examples/with-strict-csp/README.md
+++ b/examples/with-strict-csp/README.md
@@ -7,7 +7,7 @@ Note: There are still valid cases for using a nonce in case you need to inline s
## Deploy your own
-Deploy the example using [Vercel](https://vercel.com):
+Deploy the example using [Vercel](https://vercel.com?utm_source=github&utm_medium=readme&utm_campaign=next-example):
[](https://vercel.com/new/git/external?repository-url=https://github.com/vercel/next.js/tree/canary/examples/with-strict-csp&project-name=with-strict-csp&repository-name=with-strict-csp)
diff --git a/examples/with-style-sheet/README.md b/examples/with-style-sheet/README.md
index 318b354908f2d..477752559793b 100644
--- a/examples/with-style-sheet/README.md
+++ b/examples/with-style-sheet/README.md
@@ -4,7 +4,7 @@ This example features an app using the [style-sheet](https://www.npmjs.com/packa
## Deploy your own
-Deploy the example using [Vercel](https://vercel.com):
+Deploy the example using [Vercel](https://vercel.com?utm_source=github&utm_medium=readme&utm_campaign=next-example):
[](https://vercel.com/new/git/external?repository-url=https://github.com/vercel/next.js/tree/canary/examples/with-style-sheet&project-name=with-style-sheet&repository-name=with-style-sheet)
diff --git a/examples/with-styled-components-rtl/README.md b/examples/with-styled-components-rtl/README.md
index 8f23ccf186d7d..7c42db9f2e369 100644
--- a/examples/with-styled-components-rtl/README.md
+++ b/examples/with-styled-components-rtl/README.md
@@ -4,7 +4,7 @@ This example shows how to use nextjs with right to left (RTL) styles using style
## Deploy your own
-Deploy the example using [Vercel](https://vercel.com):
+Deploy the example using [Vercel](https://vercel.com?utm_source=github&utm_medium=readme&utm_campaign=next-example):
[](https://vercel.com/new/git/external?repository-url=https://github.com/vercel/next.js/tree/canary/examples/with-styled-components-rtl&project-name=with-styled-components-rtl&repository-name=with-styled-components-rtl)
diff --git a/examples/with-styled-components/README.md b/examples/with-styled-components/README.md
index 4850caa04edc8..7c585b998bb97 100644
--- a/examples/with-styled-components/README.md
+++ b/examples/with-styled-components/README.md
@@ -6,7 +6,7 @@ For this purpose we are extending the `` and injecting the server si
## Deploy your own
-Deploy the example using [Vercel](https://vercel.com):
+Deploy the example using [Vercel](https://vercel.com?utm_source=github&utm_medium=readme&utm_campaign=next-example):
[](https://vercel.com/new/git/external?repository-url=https://github.com/vercel/next.js/tree/canary/examples/with-styled-components&project-name=with-styled-components&repository-name=with-styled-components)
diff --git a/examples/with-styled-jsx-plugins/README.md b/examples/with-styled-jsx-plugins/README.md
index 3b46dae428ff0..8120988b168f2 100644
--- a/examples/with-styled-jsx-plugins/README.md
+++ b/examples/with-styled-jsx-plugins/README.md
@@ -10,7 +10,7 @@ More details about how plugins work can be found in the [styled-jsx readme](http
## Deploy your own
-Deploy the example using [Vercel](https://vercel.com):
+Deploy the example using [Vercel](https://vercel.com?utm_source=github&utm_medium=readme&utm_campaign=next-example):
[](https://vercel.com/new/git/external?repository-url=https://github.com/vercel/next.js/tree/canary/examples/with-styled-jsx-plugins&project-name=with-styled-jsx-plugins&repository-name=with-styled-jsx-plugins)
diff --git a/examples/with-styled-jsx-scss/README.md b/examples/with-styled-jsx-scss/README.md
index c06eddbafc16b..4b6323170c9a8 100644
--- a/examples/with-styled-jsx-scss/README.md
+++ b/examples/with-styled-jsx-scss/README.md
@@ -10,7 +10,7 @@ More details about how plugins work can be found in the [styled-jsx readme](http
## Deploy your own
-Deploy the example using [Vercel](https://vercel.com):
+Deploy the example using [Vercel](https://vercel.com?utm_source=github&utm_medium=readme&utm_campaign=next-example):
[](https://vercel.com/new/git/external?repository-url=https://github.com/vercel/next.js/tree/canary/examples/with-styled-jsx-scss&project-name=with-styled-jsx-scss&repository-name=with-styled-jsx-scss)
diff --git a/examples/with-styled-jsx/README.md b/examples/with-styled-jsx/README.md
index e30bf84bc6cde..f9e293bdb5451 100644
--- a/examples/with-styled-jsx/README.md
+++ b/examples/with-styled-jsx/README.md
@@ -4,7 +4,7 @@ Next.js ships with [styled-jsx](https://github.com/vercel/styled-jsx) allowing y
## Deploy your own
-Deploy the example using [Vercel](https://vercel.com):
+Deploy the example using [Vercel](https://vercel.com?utm_source=github&utm_medium=readme&utm_campaign=next-example):
[](https://vercel.com/new/git/external?repository-url=https://github.com/vercel/next.js/tree/canary/examples/with-styled-jsx&project-name=with-styled-jsx&repository-name=with-styled-jsx)
diff --git a/examples/with-styletron/README.md b/examples/with-styletron/README.md
index 458eadb1e4a8c..e42d60adca5ad 100644
--- a/examples/with-styletron/README.md
+++ b/examples/with-styletron/README.md
@@ -6,7 +6,7 @@ For this purpose we are extending the `` and injecting the server si
## Deploy your own
-Deploy the example using [Vercel](https://vercel.com):
+Deploy the example using [Vercel](https://vercel.com?utm_source=github&utm_medium=readme&utm_campaign=next-example):
[](https://vercel.com/new/git/external?repository-url=https://github.com/vercel/next.js/tree/canary/examples/with-styletron&project-name=with-styletron&repository-name=with-styletron)
diff --git a/examples/with-tailwindcss-emotion/README.md b/examples/with-tailwindcss-emotion/README.md
index b23349a83e081..42927fe9e5781 100644
--- a/examples/with-tailwindcss-emotion/README.md
+++ b/examples/with-tailwindcss-emotion/README.md
@@ -6,7 +6,7 @@ This is an example of how you can add [tailwind CSS](https://tailwindcss.com/) w
## Deploy your own
-Deploy the example using [Vercel](https://vercel.com):
+Deploy the example using [Vercel](https://vercel.com?utm_source=github&utm_medium=readme&utm_campaign=next-example):
[](https://vercel.com/new/git/external?repository-url=https://github.com/vercel/next.js/tree/canary/examples/with-tailwindcss-emotion&project-name=with-tailwindcss-emotion&repository-name=with-tailwindcss-emotion)
diff --git a/examples/with-tailwindcss/README.md b/examples/with-tailwindcss/README.md
index 06c729ea5bbee..78a54ca5d6537 100644
--- a/examples/with-tailwindcss/README.md
+++ b/examples/with-tailwindcss/README.md
@@ -4,7 +4,7 @@ This example is a basic starting point for using [Tailwind CSS](https://tailwind
## Deploy your own
-Deploy the example using [Vercel](https://vercel.com):
+Deploy the example using [Vercel](https://vercel.com?utm_source=github&utm_medium=readme&utm_campaign=next-example):
[](https://vercel.com/new/git/external?repository-url=https://github.com/vercel/next.js/tree/canary/examples/with-tailwindcss&project-name=with-tailwindcss&repository-name=with-tailwindcss)
diff --git a/examples/with-tesfy/README.md b/examples/with-tesfy/README.md
index bc69a37639d24..bb55e121ce334 100644
--- a/examples/with-tesfy/README.md
+++ b/examples/with-tesfy/README.md
@@ -12,7 +12,7 @@ The `datafile` is just a `json` that defines the configuration of the experiment
## Deploy your own
-Deploy the example using [Vercel](https://vercel.com):
+Deploy the example using [Vercel](https://vercel.com?utm_source=github&utm_medium=readme&utm_campaign=next-example):
[](https://vercel.com/new/git/external?repository-url=https://github.com/vercel/next.js/tree/canary/examples/with-tesfy&project-name=with-tesfy&repository-name=with-tesfy)
diff --git a/examples/with-three-js/README.md b/examples/with-three-js/README.md
index 183b010f9a954..c22d4774c92fd 100644
--- a/examples/with-three-js/README.md
+++ b/examples/with-three-js/README.md
@@ -8,7 +8,7 @@ This example uses:
## Deploy your own
-Deploy the example using [Vercel](https://vercel.com):
+Deploy the example using [Vercel](https://vercel.com?utm_source=github&utm_medium=readme&utm_campaign=next-example):
[](https://vercel.com/new/git/external?repository-url=https://github.com/vercel/next.js/tree/canary/examples/with-three-js&project-name=with-three-js&repository-name=with-three-js)
diff --git a/examples/with-typescript-eslint-jest/README.md b/examples/with-typescript-eslint-jest/README.md
index ed2c02e786a5d..9f84531ec4916 100644
--- a/examples/with-typescript-eslint-jest/README.md
+++ b/examples/with-typescript-eslint-jest/README.md
@@ -10,7 +10,7 @@ Bootstrap a developer-friendly NextJS app configured with:
## Deploy your own
-Deploy the example using [Vercel](https://vercel.com):
+Deploy the example using [Vercel](https://vercel.com?utm_source=github&utm_medium=readme&utm_campaign=next-example):
[](https://vercel.com/new/git/external?repository-url=https://github.com/vercel/next.js/tree/canary/examples/with-typescript-eslint-jest&project-name=with-typescript-eslint-jest&repository-name=with-typescript-eslint-jest)
diff --git a/examples/with-typescript-graphql/README.md b/examples/with-typescript-graphql/README.md
index 46ec84f423b31..fa868934555bc 100644
--- a/examples/with-typescript-graphql/README.md
+++ b/examples/with-typescript-graphql/README.md
@@ -19,7 +19,7 @@ By default `**/*.graphqls` is recognized as GraphQL schema and `**/*.graphql` as
## Deploy your own
-Deploy the example using [Vercel](https://vercel.com):
+Deploy the example using [Vercel](https://vercel.com?utm_source=github&utm_medium=readme&utm_campaign=next-example):
[](https://vercel.com/new/git/external?repository-url=https://github.com/vercel/next.js/tree/canary/examples/with-typescript-graphql&project-name=with-typescript-graphql&repository-name=with-typescript-graphql)
diff --git a/examples/with-typescript-styled-components/README.md b/examples/with-typescript-styled-components/README.md
index c7fc97ed9ecc4..dbb22d0b9e3b6 100644
--- a/examples/with-typescript-styled-components/README.md
+++ b/examples/with-typescript-styled-components/README.md
@@ -4,7 +4,7 @@ This is a really simple project that show the usage of Next.js with TypeScript a
## Deploy your own
-Deploy the example using [Vercel](https://vercel.com):
+Deploy the example using [Vercel](https://vercel.com?utm_source=github&utm_medium=readme&utm_campaign=next-example):
[](https://vercel.com/new/git/external?repository-url=https://github.com/vercel/next.js/tree/canary/examples/with-typescript-styled-components&project-name=with-typescript-styled-components&repository-name=with-typescript-styled-components)
diff --git a/examples/with-typescript/README.md b/examples/with-typescript/README.md
index 4222f81d9d2f4..514bf1de55608 100644
--- a/examples/with-typescript/README.md
+++ b/examples/with-typescript/README.md
@@ -4,7 +4,7 @@ This is a really simple project that shows the usage of Next.js with TypeScript.
## Deploy your own
-Deploy the example using [Vercel](https://vercel.com):
+Deploy the example using [Vercel](https://vercel.com?utm_source=github&utm_medium=readme&utm_campaign=next-example):
[](https://vercel.com/new/git/external?repository-url=https://github.com/vercel/next.js/tree/canary/examples/with-typescript&project-name=with-typescript&repository-name=with-typescript)
diff --git a/examples/with-typestyle/README.md b/examples/with-typestyle/README.md
index 6a0b3b558a761..5624d5c29796c 100644
--- a/examples/with-typestyle/README.md
+++ b/examples/with-typestyle/README.md
@@ -6,7 +6,7 @@ For this purpose we are extending the `` and injecting the server si
## Deploy your own
-Deploy the example using [Vercel](https://vercel.com):
+Deploy the example using [Vercel](https://vercel.com?utm_source=github&utm_medium=readme&utm_campaign=next-example):
[](https://vercel.com/new/git/external?repository-url=https://github.com/vercel/next.js/tree/canary/examples/with-typestyle&project-name=with-typestyle&repository-name=with-typestyle)
diff --git a/examples/with-unstated/README.md b/examples/with-unstated/README.md
index 7b7beb035d280..d1abf33f1e3a5 100644
--- a/examples/with-unstated/README.md
+++ b/examples/with-unstated/README.md
@@ -6,7 +6,7 @@ There are two pages, `/` and `/about`, both render a counter and a timer, the st
## Deploy your own
-Deploy the example using [Vercel](https://vercel.com):
+Deploy the example using [Vercel](https://vercel.com?utm_source=github&utm_medium=readme&utm_campaign=next-example):
[](https://vercel.com/new/git/external?repository-url=https://github.com/vercel/next.js/tree/canary/examples/with-unstated&project-name=with-unstated&repository-name=with-unstated)
diff --git a/examples/with-videojs/README.md b/examples/with-videojs/README.md
index 596c4799c3555..58cf100cd7413 100644
--- a/examples/with-videojs/README.md
+++ b/examples/with-videojs/README.md
@@ -4,7 +4,7 @@ This example shows how to use Next.js along with [Video.js](https://videojs.com)
## Deploy your own
-Deploy the example using [Vercel](https://vercel.com):
+Deploy the example using [Vercel](https://vercel.com?utm_source=github&utm_medium=readme&utm_campaign=next-example):
[](https://vercel.com/new/git/external?repository-url=https://github.com/vercel/next.js/tree/canary/examples/with-videojs&project-name=with-videojs&repository-name=with-videojs)
diff --git a/examples/with-why-did-you-render/README.md b/examples/with-why-did-you-render/README.md
index b394473776700..f7816fcb942ad 100644
--- a/examples/with-why-did-you-render/README.md
+++ b/examples/with-why-did-you-render/README.md
@@ -33,7 +33,7 @@ You can see `why-did-you-render` console logs about this redundant re-render in
## Deploy your own
-Deploy the example using [Vercel](https://vercel.com):
+Deploy the example using [Vercel](https://vercel.com?utm_source=github&utm_medium=readme&utm_campaign=next-example):
[](https://vercel.com/new/git/external?repository-url=https://github.com/vercel/next.js/tree/canary/examples/with-why-did-you-render&project-name=with-why-did-you-render&repository-name=with-why-did-you-render)
diff --git a/examples/with-xstate/README.md b/examples/with-xstate/README.md
index decbacc3ff3cf..f9e2e827efe13 100644
--- a/examples/with-xstate/README.md
+++ b/examples/with-xstate/README.md
@@ -4,7 +4,7 @@ This example shows how to integrate XState in Next.js. For more info about XStat
## Deploy your own
-Deploy the example using [Vercel](https://vercel.com):
+Deploy the example using [Vercel](https://vercel.com?utm_source=github&utm_medium=readme&utm_campaign=next-example):
[](https://vercel.com/new/git/external?repository-url=https://github.com/vercel/next.js/tree/canary/examples/with-xstate&project-name=with-xstate&repository-name=with-xstate)
diff --git a/examples/with-yarn-workspaces/README.md b/examples/with-yarn-workspaces/README.md
index c3e7ed930164d..59d8d669e3b53 100644
--- a/examples/with-yarn-workspaces/README.md
+++ b/examples/with-yarn-workspaces/README.md
@@ -10,7 +10,7 @@ In this example we have three workspaces:
## Deploy your own
-Deploy the example using [Vercel](https://vercel.com):
+Deploy the example using [Vercel](https://vercel.com?utm_source=github&utm_medium=readme&utm_campaign=next-example):
[](https://vercel.com/new/git/external?repository-url=https://github.com/vercel/next.js/tree/canary/examples/with-yarn-workspaces&project-name=with-yarn-workspaces&repository-name=with-yarn-workspaces)
diff --git a/examples/with-zeit-fetch/README.md b/examples/with-zeit-fetch/README.md
index dfbea9fe93612..647b7222eb688 100644
--- a/examples/with-zeit-fetch/README.md
+++ b/examples/with-zeit-fetch/README.md
@@ -4,7 +4,7 @@ This example shows how to use [`@zeit/fetch`](https://npmjs.com/package/@zeit/fe
## Deploy your own
-Deploy the example using [Vercel](https://vercel.com):
+Deploy the example using [Vercel](https://vercel.com?utm_source=github&utm_medium=readme&utm_campaign=next-example):
[](https://vercel.com/new/git/external?repository-url=https://github.com/vercel/next.js/tree/canary/examples/with-zeit-fetch&project-name=with-zeit-fetch&repository-name=with-zeit-fetch)
diff --git a/examples/with-zustand/README.md b/examples/with-zustand/README.md
index e650d0d16a959..fcfa14abbf05a 100644
--- a/examples/with-zustand/README.md
+++ b/examples/with-zustand/README.md
@@ -16,7 +16,7 @@ On the server side every request initializes a new store, because otherwise diff
## Deploy your own
-Deploy the example using [Vercel](https://vercel.com):
+Deploy the example using [Vercel](https://vercel.com?utm_source=github&utm_medium=readme&utm_campaign=next-example):
[](https://vercel.com/new/git/external?repository-url=https://github.com/vercel/next.js/tree/canary/examples/with-zustand&project-name=with-zustand&repository-name=with-zustand)
From 85624d6164a043959ed5d29e94fce7207b15fbbb Mon Sep 17 00:00:00 2001
From: Tim Neutkens
Date: Tue, 19 Jan 2021 09:44:03 +0100
Subject: [PATCH 4/5] Remove console.log
---
packages/next/build/webpack/plugins/profiling-plugin.ts | 4 ----
1 file changed, 4 deletions(-)
diff --git a/packages/next/build/webpack/plugins/profiling-plugin.ts b/packages/next/build/webpack/plugins/profiling-plugin.ts
index 6dee491cd8499..908164d6aa5d6 100644
--- a/packages/next/build/webpack/plugins/profiling-plugin.ts
+++ b/packages/next/build/webpack/plugins/profiling-plugin.ts
@@ -18,10 +18,6 @@ export class ProfilingPlugin {
apply(compiler: any) {
// Only enabled when instrumentation is loaded
const currentSpan = tracer.getCurrentSpan()
- console.log({
- currentSpan,
- isRecording: currentSpan?.isRecording(),
- })
if (!currentSpan || !currentSpan.isRecording()) {
return
}
From 0bd175264b000895cccd75f4499bdf55daad4882 Mon Sep 17 00:00:00 2001
From: =?UTF-8?q?Thor=20=E9=9B=B7=E7=A5=9E=20Schaeff?=
<5748289+thorwebdev@users.noreply.github.com>
Date: Tue, 19 Jan 2021 21:10:56 +0800
Subject: [PATCH 5/5] Update Supabase example. (#21200)
---
.../.env.local.example | 3 +-
.../with-supabase-auth-realtime-db/README.md | 144 ++------------
.../components/Layout.js | 80 --------
.../components/Message.js | 10 -
.../components/MessageInput.js | 28 ---
.../docs/quickstart.png | Bin 104918 -> 0 bytes
.../docs/slack-clone-demo.gif | Bin 1607450 -> 0 bytes
.../jsconfig.json | 8 -
.../lib/Store.js | 169 ----------------
.../lib/UserContext.js | 5 -
.../package.json | 18 +-
.../pages/_app.js | 80 ++------
.../pages/api/auth.js | 8 +
.../pages/api/getUser.js | 13 ++
.../pages/channels/[id].js | 53 -----
.../pages/index.js | 182 +++++++++++-------
.../pages/profile.js | 41 ++++
.../postcss.config.js | 6 -
.../with-supabase-auth-realtime-db/style.css | 4 +
.../styles/style.scss | 28 ---
.../tailwind.config.js | 11 --
.../utils/initSupabase.js | 6 +
22 files changed, 213 insertions(+), 684 deletions(-)
delete mode 100644 examples/with-supabase-auth-realtime-db/components/Layout.js
delete mode 100644 examples/with-supabase-auth-realtime-db/components/Message.js
delete mode 100644 examples/with-supabase-auth-realtime-db/components/MessageInput.js
delete mode 100644 examples/with-supabase-auth-realtime-db/docs/quickstart.png
delete mode 100644 examples/with-supabase-auth-realtime-db/docs/slack-clone-demo.gif
delete mode 100644 examples/with-supabase-auth-realtime-db/jsconfig.json
delete mode 100644 examples/with-supabase-auth-realtime-db/lib/Store.js
delete mode 100644 examples/with-supabase-auth-realtime-db/lib/UserContext.js
create mode 100644 examples/with-supabase-auth-realtime-db/pages/api/auth.js
create mode 100644 examples/with-supabase-auth-realtime-db/pages/api/getUser.js
delete mode 100644 examples/with-supabase-auth-realtime-db/pages/channels/[id].js
create mode 100644 examples/with-supabase-auth-realtime-db/pages/profile.js
delete mode 100644 examples/with-supabase-auth-realtime-db/postcss.config.js
create mode 100644 examples/with-supabase-auth-realtime-db/style.css
delete mode 100644 examples/with-supabase-auth-realtime-db/styles/style.scss
delete mode 100644 examples/with-supabase-auth-realtime-db/tailwind.config.js
create mode 100644 examples/with-supabase-auth-realtime-db/utils/initSupabase.js
diff --git a/examples/with-supabase-auth-realtime-db/.env.local.example b/examples/with-supabase-auth-realtime-db/.env.local.example
index b6fedec92e308..477da3d401d67 100644
--- a/examples/with-supabase-auth-realtime-db/.env.local.example
+++ b/examples/with-supabase-auth-realtime-db/.env.local.example
@@ -1,2 +1,3 @@
+# Update these with your Supabase details from your project settings > API
NEXT_PUBLIC_SUPABASE_URL=https://your-project.supabase.co
-NEXT_PUBLIC_SUPABASE_KEY=your-anon-key
\ No newline at end of file
+NEXT_PUBLIC_SUPABASE_ANON_KEY=your-anon-key
\ No newline at end of file
diff --git a/examples/with-supabase-auth-realtime-db/README.md b/examples/with-supabase-auth-realtime-db/README.md
index 537029ce5f8d5..0f64a1dc7140f 100644
--- a/examples/with-supabase-auth-realtime-db/README.md
+++ b/examples/with-supabase-auth-realtime-db/README.md
@@ -1,139 +1,21 @@
-# Realtime chat example using Supabase
+# Example: [Supabase authentication](https://supabase.io/docs/guides/auth) client- and server-side (API routes), and SSR with auth cookie.
-This is a full-stack Slack clone example using:
+This example shows how to use Supabase auth on the client and server in both [API routes](https://nextjs.org/docs/api-routes/introduction) and when using [server side rendering (SSR)](https://nextjs.org/docs/basic-features/pages#server-side-rendering).
-- Frontend:
- - Next.js.
- - [Supabase](https://supabase.io/docs/library/getting-started) for user management and realtime data syncing.
-- Backend:
- - [app.supabase.io](https://app.supabase.io/): hosted Postgres database with restful API for usage with Supabase.js.
+## Deploy with Vercel
-
+The Vercel deployment will guide you through creating a Supabase account and project. After installation of the Supabase integration, all relevant environment variables will be set up so that the project is usable immediately after deployment 🚀
-This example is a clone of the [Slack Clone example](https://github.com/supabase/supabase/tree/master/examples/nextjs-slack-clone) in the supabase repo, feel free to check it out!
+[](https://vercel.com/new/git/external?repository-url=https%3A%2F%2Fgithub.com%2Fsupabase%2Fsupabase%2Ftree%2Fmaster%2Fexamples%2Fnextjs-with-supabase-auth&project-name=nextjs-with-supabase-auth&repository-name=nextjs-with-supabase-auth&integration-ids=oac_jUduyjQgOyzev1fjrW83NYOv)
-## Deploy your own
+## Feedback and issues
-Once you have access to [the environment variables you'll need](#step-3-set-up-environment-variables), deploy the example using [Vercel](https://vercel.com?utm_source=github&utm_medium=readme&utm_campaign=next-example):
+Please file feedback and issues over on the [Supabase GitHub org](https://github.com/supabase/supabase/issues/new/choose).
-[](https://vercel.com/new/git/external?repository-url=https://github.com/vercel/next.js/tree/canary/examples/with-supabase-auth-realtime-db&project-name=with-supabase-auth-realtime-db&repository-name=with-supabase-auth-realtime-db&env=NEXT_PUBLIC_SUPABASE_URL,NEXT_PUBLIC_SUPABASE_KEY&envDescription=Required%20to%20connect%20the%20app%to%Supabase&envLink=https://github.com/vercel/next.js/tree/canary/examples/with-supabase-auth-realtime-db%23step-3-set-up-environment-variables&project-name=supabase-slack-clone)
+## More Supabase examples
-## How to use
-
-Execute [`create-next-app`](https://github.com/vercel/next.js/tree/canary/packages/create-next-app) with [npm](https://docs.npmjs.com/cli/init) or [Yarn](https://yarnpkg.com/lang/en/docs/cli/create/) to bootstrap the example:
-
-```bash
-npx create-next-app --example with-supabase-auth-realtime-db realtime-chat-app
-# or
-yarn create next-app --example with-supabase-auth-realtime-db realtime-chat-app
-```
-
-## Configuration
-
-### Step 1. Create a new Supabase project
-
-Sign up to Supabase - [https://app.supabase.io](https://app.supabase.io) and create a new project. Wait for your database to start.
-
-### Step 2. Run the "Slack Clone" Quickstart
-
-Once your database has started, run the "Slack Clone" quickstart.
-
-
-
-### Step 3. Set up environment variables
-
-In your Supabase project, go to Project Settings (the cog icon), open the API tab, and find your **API URL** and **anon** key, you'll need these in the next step.
-
-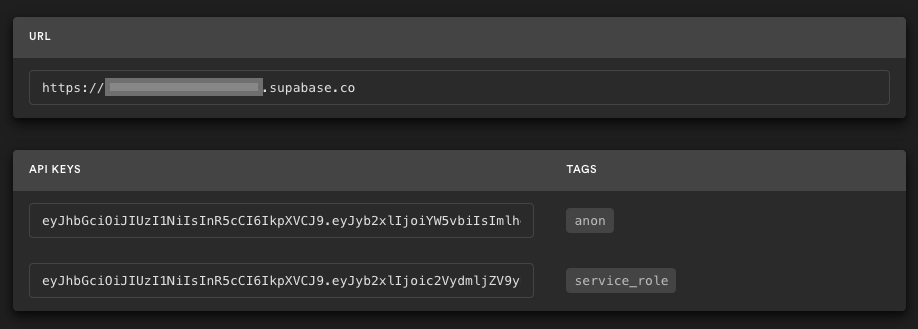
-
-Next, copy the `.env.local.example` file in this directory to `.env.local` (which will be ignored by Git):
-
-```bash
-cp .env.local.example .env.local
-```
-
-Then set each variable on `.env.local`:
-
-- `NEXT_PUBLIC_SUPABASE_URL` should be the **API URL**
-- `NEXT_PUBLIC_SUPABASE_KEY` should be the **anon** key
-
-The **anon** key is your client-side API key. It allows "anonymous access" to your database, until the user has logged in. Once they have logged in, the keys will switch to the user's own login token. This enables row level security for your data. Read more about this [below](#postgres-row-level-security).
-
-> **_NOTE_**: The `service_role` key has full access to your data, bypassing any security policies. These keys have to be kept secret and are meant to be used in server environments and never on a client or browser.
-
-### Step 4. Run Next.js in development mode
-
-```bash
-npm install
-npm run dev
-
-# or
-
-yarn install
-yarn dev
-```
-
-Visit [http://localhost:3000](http://localhost:3000) and start chatting! Open a channel across two browser tabs to see everything getting updated in realtime 🥳. If it doesn't work, post on [GitHub discussions](https://github.com/vercel/next.js/discussions).
-
-### Step 5. Deploy on Vercel
-
-You can deploy this app to the cloud with [Vercel](https://vercel.com?utm_source=github&utm_medium=readme&utm_campaign=next-example) ([Documentation](https://nextjs.org/docs/deployment)).
-
-#### Deploy Your Local Project
-
-To deploy your local project to Vercel, push it to GitHub/GitLab/Bitbucket and [import to Vercel](https://vercel.com/new?utm_source=github&utm_medium=readme&utm_campaign=next-example).
-
-**Important**: When you import your project on Vercel, make sure to click on **Environment Variables** and set them to match your `.env.local` file.
-
-#### Deploy from Our Template
-
-Alternatively, you can deploy using our template by clicking on the Deploy button below.
-
-[](https://vercel.com/new/git/external?repository-url=https://github.com/vercel/next.js/tree/canary/examples/with-supabase-auth-realtime-db&project-name=with-supabase-auth-realtime-db&repository-name=with-supabase-auth-realtime-db&env=NEXT_PUBLIC_SUPABASE_URL,NEXT_PUBLIC_SUPABASE_KEY&envDescription=Required%20to%20connect%20the%20app%to%Supabase&envLink=https://github.com/vercel/next.js/tree/canary/examples/with-supabase-auth-realtime-db%23step-3-set-up-environment-variables&project-name=supabase-slack-clone)
-
-## Supabase details
-
-### Postgres Row level security
-
-This project uses very high-level Authorization using Postgres' Role Level Security.
-When you start a Postgres database on Supabase, we populate it with an `auth` schema, and some helper functions.
-When a user logs in, they are issued a JWT with the role `authenticated` and thier UUID.
-We can use these details to provide fine-grained control over what each user can and cannot do.
-
-This is a trimmed-down schema, with the policies:
-
-```sql
--- USER PROFILES
-CREATE TYPE public.user_status AS ENUM ('ONLINE', 'OFFLINE');
-CREATE TABLE public.users (
- id uuid NOT NULL PRIMARY KEY, -- UUID from auth.users (Supabase)
- username text,
- status user_status DEFAULT 'OFFLINE'::public.user_status
-);
-ALTER TABLE public.users ENABLE ROW LEVEL SECURITY;
-CREATE POLICY "Allow logged-in read access" on public.users FOR SELECT USING ( auth.role() = 'authenticated' );
-CREATE POLICY "Allow individual insert access" on public.users FOR INSERT WITH CHECK ( auth.uid() = id );
-CREATE POLICY "Allow individual update access" on public.users FOR UPDATE USING ( auth.uid() = id );
-
--- CHANNELS
-CREATE TABLE public.channels (
- id bigint GENERATED BY DEFAULT AS IDENTITY PRIMARY KEY,
- inserted_at timestamp with time zone DEFAULT timezone('utc'::text, now()) NOT NULL,
- slug text NOT NULL UNIQUE
-);
-ALTER TABLE public.channels ENABLE ROW LEVEL SECURITY;
-CREATE POLICY "Allow logged-in full access" on public.channels FOR ALL USING ( auth.role() = 'authenticated' );
-
--- MESSAGES
-CREATE TABLE public.messages (
- id bigint GENERATED BY DEFAULT AS IDENTITY PRIMARY KEY,
- inserted_at timestamp with time zone DEFAULT timezone('utc'::text, now()) NOT NULL,
- message text,
- user_id uuid REFERENCES public.users NOT NULL,
- channel_id bigint REFERENCES public.channels NOT NULL
-);
-ALTER TABLE public.messages ENABLE ROW LEVEL SECURITY;
-CREATE POLICY "Allow logged-in read access" on public.messages USING ( auth.role() = 'authenticated' );
-CREATE POLICY "Allow individual insert access" on public.messages FOR INSERT WITH CHECK ( auth.uid() = user_id );
-CREATE POLICY "Allow individual update access" on public.messages FOR UPDATE USING ( auth.uid() = user_id );
-```
+- [Next.js Subscription Payments Starter](https://github.com/vercel/nextjs-subscription-payments)
+- [Next.js Slack Clone](https://github.com/supabase/supabase/tree/master/examples/nextjs-slack-clone)
+- [Next.js Todo List](https://github.com/supabase/supabase/tree/master/examples/nextjs-todo-list)
+- [Next.js Live Tracker Map](https://github.com/supabase/supabase/tree/master/examples/nextjs-live-tracker-map)
+- [And many more...](https://github.com/supabase/supabase/tree/master/examples)
diff --git a/examples/with-supabase-auth-realtime-db/components/Layout.js b/examples/with-supabase-auth-realtime-db/components/Layout.js
deleted file mode 100644
index 1b94dea575aa5..0000000000000
--- a/examples/with-supabase-auth-realtime-db/components/Layout.js
+++ /dev/null
@@ -1,80 +0,0 @@
-import Link from 'next/link'
-import { useContext } from 'react'
-import UserContext from '~/lib/UserContext'
-import { addChannel } from '~/lib/Store'
-
-export default function Layout(props) {
- const { signOut } = useContext(UserContext)
-
- const slugify = (text) => {
- return text
- .toString()
- .toLowerCase()
- .replace(/\s+/g, '-') // Replace spaces with -
- .replace(/[^\w-]+/g, '') // Remove all non-word chars
- .replace(/--+/g, '-') // Replace multiple - with single -
- .replace(/^-+/, '') // Trim - from start of text
- .replace(/-+$/, '') // Trim - from end of text
- }
-
- const newChannel = async () => {
- const slug = prompt('Please enter your name')
- if (slug) {
- addChannel(slugify(slug))
- }
- }
-
- return (
-
- {/* Sidebar */}
-
-
- {/* Messages */}
-