-
Notifications
You must be signed in to change notification settings - Fork 2.4k
New issue
Have a question about this project? Sign up for a free GitHub account to open an issue and contact its maintainers and the community.
By clicking “Sign up for GitHub”, you agree to our terms of service and privacy statement. We’ll occasionally send you account related emails.
Already on GitHub? Sign in to your account
Any plans to add support for SLIP-0132 version bytes? #1797
Comments
The cleanest approach I can think of is to provide a new method called // RegisterHDKeyID registers a public and private hierarchical deterministic
// extended key ID pair.
//
// Non-standard HD version bytes, such as the ones documented in SLIP-0132,
// should be registered using this method for library packages to lookup key
// IDs (aka HD version bytes).
// Reference:
// SLIP-0132 : Registered HD version bytes for BIP-0032
// https://github.com/satoshilabs/slips/blob/master/slip-0132.md
func RegisterHDKeyID(hdPublicKeyID []byte, hdPrivateKeyID []byte) error {
if len(hdPublicKeyID) != 4 || len(hdPrivateKeyID) != 4 {
return ErrInvalidHDKeyID
}
var keyID [4]byte
copy(keyID[:], hdPrivateKeyID)
hdPrivToPubKeyIDs[keyID] = hdPublicKeyID
return nil
} Using the above solution, you don't need to modify the hdKeyIDZprv := []byte{0x02, 0xaa, 0x7a, 0x99}
hdKeyIDZpub := []byte{0x02, 0xaa, 0x7e, 0xd3}
if err := chaincfg.RegisterHDKeyID(hdKeyIDZprv, hdKeyIDZpub); err != nil {
panic(err)
}
masterZpub, err = masterZprv.Neuter() // assuming masterZprv is already defined
if err != nil {
panic(err)
}
fmt.Println("serialized master Zpub", masterZpub.String()) // this should work! I can do a PR if the above works for you. |
That makes sense, thanks! Would it be easy to switch version bytes on an existing
You can see there's some demand for step 3 as there exists a popular tool just to perform this step: If it's not easy to switch version bytes on an already-derived child key, I could still make this work in a hackey way: just do step 1 n times (once for each version byte I'd like to encode with), perform step 2 on each of those keys, and then calculate the |
That's quite useful indeed. I don't think it's too hard to implement this feature. We just need to export the key, err := hdkeychain.NewKeyFromString("xpub67uA5wAUuv1ypp7rEY7jUZBZmwFSULFUArLBJrHr3amnymkUEYWzQJz13zLacZv33sSuxKVmerpZeFExapBNt8HpAqtTtWqDQRAgyqSKUHu")
if err != nil {
panic(err)
}
key.Version = []byte{0x04, 0xb2, 0x47, 0x46} // set the Version field for zpub from SLIP-0132
fmt.Println(key) // zpub6mZghGWKDH6wXQW5uFgytjNa7sYLMaEU15Ncse5cobXZ5yNvjrr7eSJH6QFkcPDss9gXTGgtaBXfQpU62D1QUbf1uXHK4LUBwsHykyMwZ3t I'll try to consolidate what we discussed in this issue, and do some PRs soon. :) |
That sounds awesome! I want to open-source a CLI multisig and BIP39 RNG helper tool I've written which currently uses Nit/Q: you're using
|
@mflaxman Sorry for the late response. I am looking forward to your multisig tool. 🙂
The approach discussed here is indeed cleaner. The main issue with your original implementation was that you were modifying the
You can find below a pseudo-code for the above. Let me know if this is what you want. xprv, _ := hdkeychain.NewKeyFromString("xprv...") // load the private extended key from a string
xpub, _ := xprv.Neuter() // gives you the xpub
xprv.Version = []byte{0x04, 0xb2, 0x43, 0x0c} // the xprv is now a zprv
xprv.String() // gives you the serialized zprv
zpub, _ = xprv.Neuter() // gives you the zpub
An It does not make sense for an |
+1 to everything you said. Looking forward to being able to use that. Very clean & easy to read. Thanks! |
@mflaxman I did two PRs to add support for this feature.
Check out the unit-tests of btcsuite/btcutil#181 for a sneak peek. |
Looks great! Nit: could you add a test of |
We'll need #1617 for that, which won't be available in a stable btcd version until v0.22.0 You should still be able to use it in your code by pointing to a development release of btcd, but I can only add a unit-test once btcd v0.22.0 is out. |
With #1617 and btcsuite/btcutil#181 merged, we now have a flexible API to support custom HD version bytes (including I let you close the issue after verifying the recent updates. Thanks for your constant feedback. 🙂 |
Transferred from the old |
https://github.com/satoshilabs/slips/blob/master/slip-0132.md
It appears you can pass in version bytes when constructing a master key and this will work for serializing private keys, but you cannot serialize public keys.
I was able to cobble together this hackey code, is there a better way to do this? Posting in case it helps others with the same issue.
You can compare this here to confirm it is indeed correct:
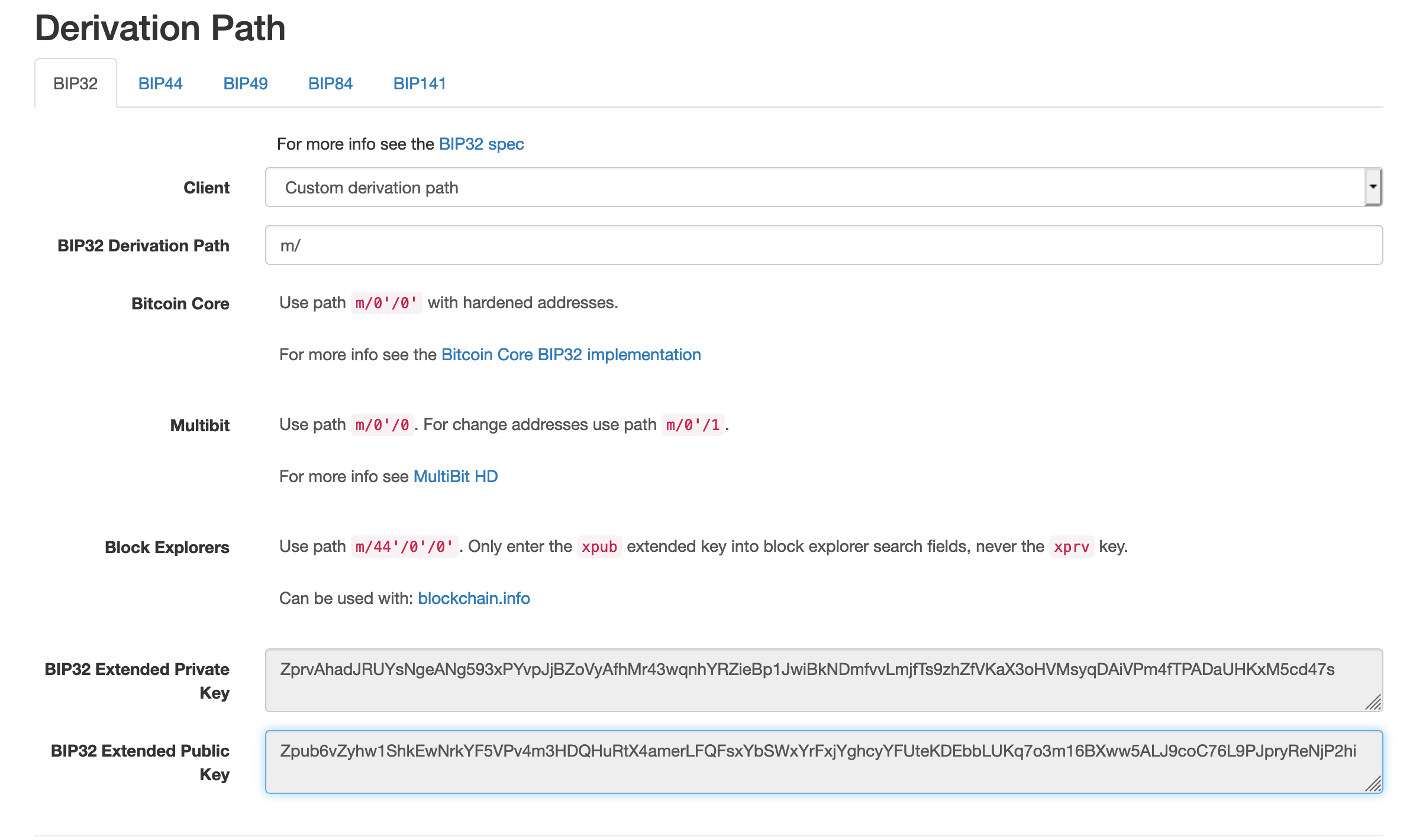
https://iancoleman.io/bip39/
Note that I'm running
github.com/btcsuite/btcutil v1.0.3-0.20200713135911-4649e4b73b34
as there were some recent changes that are not included in1.0.2
The text was updated successfully, but these errors were encountered: