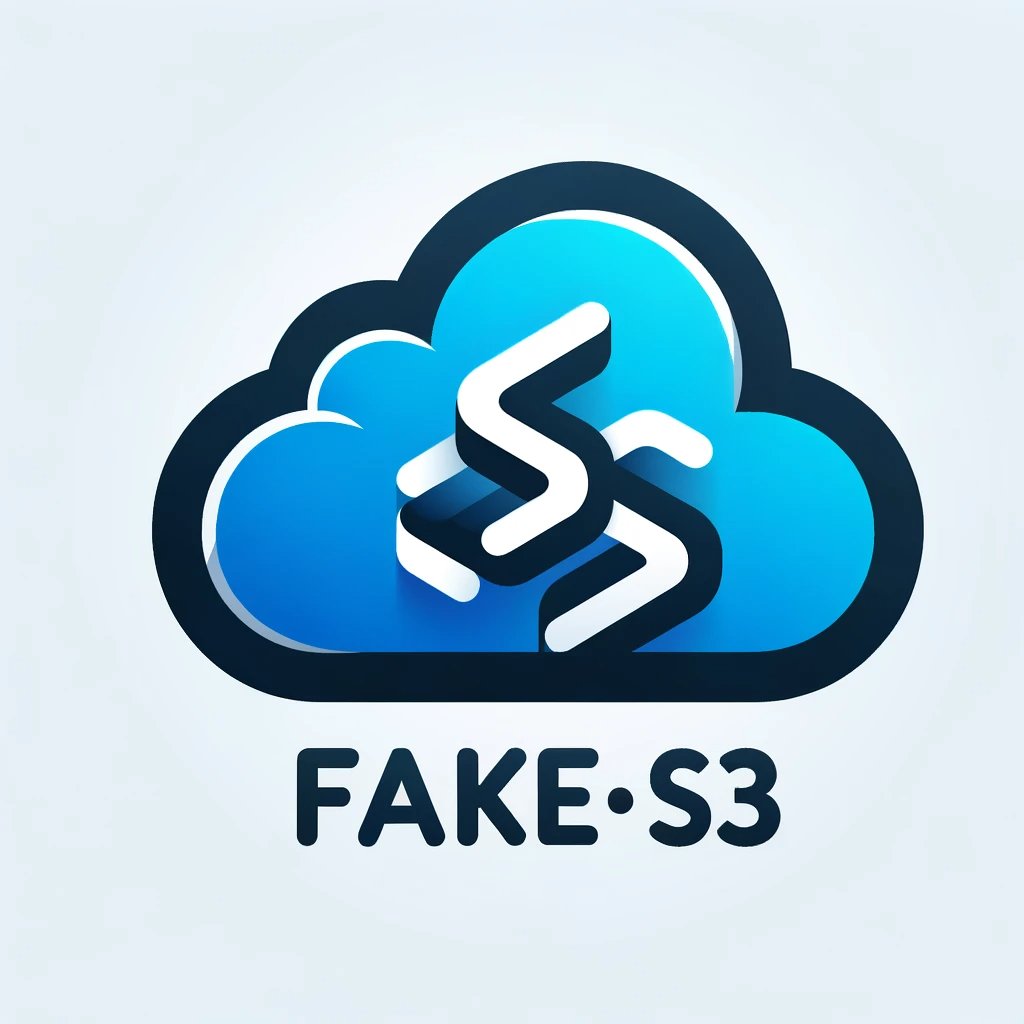
fake-s3 is a lightweight server that responds to the same API as Amazon S3.
Explore the docs »
Report Bug/Issue
·
Request Feature
fake-s3 is a lightweight server that responds to the same API as Amazon S3. It is extremely useful for testing S3 in a sandbox environment without actually making calls to Amazon, which is useful for testing code using S3 but without the cost and complications of using the real Amazon S3.
fake-s3 is built using the Go-Fake-S3 library.
Please don't use Fake S3 as a production service. The intended use case for Fake S3 is currently to facilitate testing. It's not meant to be used for safe, persistent access to production data at the moment.
To run the project locally, you will need to make sure you have configured the config.yml
correctly with the correct values for your environment. The config.yml
file is located in the conf
directory.
general:
port: 8080
read_timeout: 15
write_timeout: 15
You can run the Fake S3
as a docker container using the following commands;
-
Build the container image
docker build -t fake-s3:develop .
-
Populate the
config.yaml
file with the various values. -
Run the container
docker run -e CONFIG_PATH="./conf/" -p 8080:8080 -d --name fake-s3 fake-s3:develop
Or you can run the Fake S3
as a standalone binary using the following commands;
-
Build the binary
go mod tidy go build -o bin/fake-s3 cmd/fake-s3/main.go
-
Populate the
config.yml
file with the various values.
You can use the config.yml included in the repo as a template.
-
Run the binary
export CONFIG_PATH="./conf/" ./bin/fake-s3
// Setup a new config
cfg, _ := config.LoadDefaultConfig(
context.TODO(),
config.WithCredentialsProvider(credentials.NewStaticCredentialsProvider("KEY", "SECRET", "SESSION")),
config.WithHTTPClient(&http.Client{
Transport: &http.Transport{
TLSClientConfig: &tls.Config{InsecureSkipVerify: true},
},
}),
config.WithEndpointResolverWithOptions(
aws.EndpointResolverWithOptionsFunc(func(_, _ string, _ ...interface{}) (aws.Endpoint, error) {
return aws.Endpoint{URL: ts.URL}, nil
}),
),
)
// Create an Amazon S3 v2 client, important to use o.UsePathStyle
// alternatively change local DNS settings, e.g., in /etc/hosts
// to support requests to http://<bucketname>.127.0.0.1:32947/...
client := s3.NewFromConfig(cfg, func(o *s3.Options) {
o.UsePathStyle = true
})
// Create a bucket
client.CreateBucket(context.TODO(), &s3.CreateBucketInput{
Bucket: aws.String("fake-s3-bucket"),
})
// Upload an object
tempFile, err := os.CreateTemp(os.TempDir(), "test.txt")
if err != nil {
fmt.Println(err)
}
result, err := client.PutObject(context.TODO(), &s3.PutObjectInput{
Bucket: aws.String("fake-s3-bucket"),
Key: aws.String(filepath.Base(tempFile.Name())),
Body: tempFile,
})
if err != nil {
fmt.Println(err)
}
See the open issues for a full list of proposed features (and known issues).
Contributions are what make the open source community such an amazing place to learn, inspire, and create. Any contributions you make are greatly appreciated.
If you have a suggestion that would make this better, please fork the repo and create a pull request. You can also simply open an issue with the tag "enhancement". Don't forget to give the project a star! Thanks again!
- Clone or Fork the project
- Create your Feature Branch (
git checkout -b feature/AmazingFeature
) - Commit your Changes (
git commit -m 'Add some AmazingFeature'
) - Push to the Branch (
git push origin feature/AmazingFeature
) - Open a Pull Request to merge into develop
Distributed under the Apache License. See LICENSE.txt
for more information.
Project Link: https://github.com/sculley/fake-s3