-
-
Notifications
You must be signed in to change notification settings - Fork 140
Commit
This commit does not belong to any branch on this repository, and may belong to a fork outside of the repository.
fix(console): wrap controls line when the terminal is too narrow (#231)
Currently, the controls line for table views is never wrapped, even if it's too long to fit on a single line in the current terminal width. This isn't great...especially since PR #223 is adding more controls, and will make the line even longer. This branch allows wrapping the controls paragraph if it doesn't fit in the current area. The code for this was surprisingly complex: while `tui` supports wrapping text, layout area sizes are determined _before_ text wrapping. So, we can't just say "use as many lines as are necessary to fit this text in the current terminal width", we have to implement that logic ourselves, and pass the required number of lines to the `Layout::constraints` method. ## Screenshots On the main branch, we don't wrap. Here's a wide enough terminal: 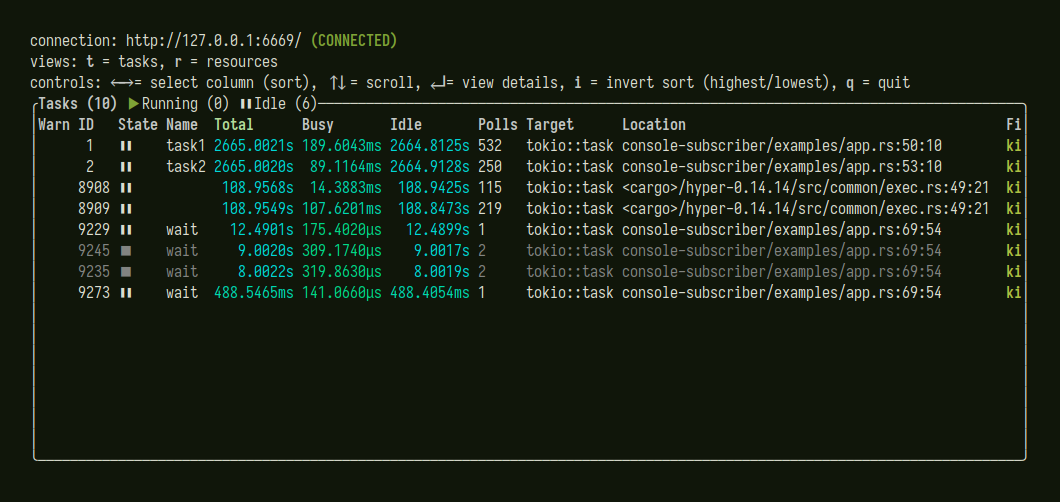 ...and here's one that's too narrow: 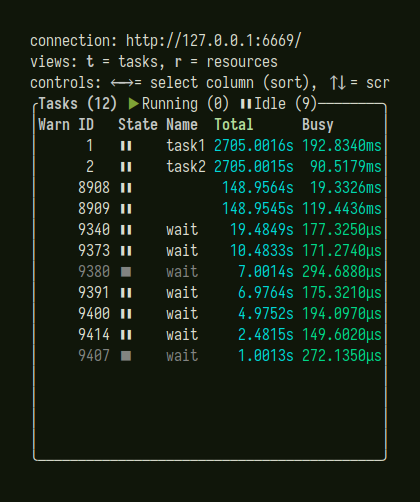 After this change, when the terminal is wide enough, it looks the same: 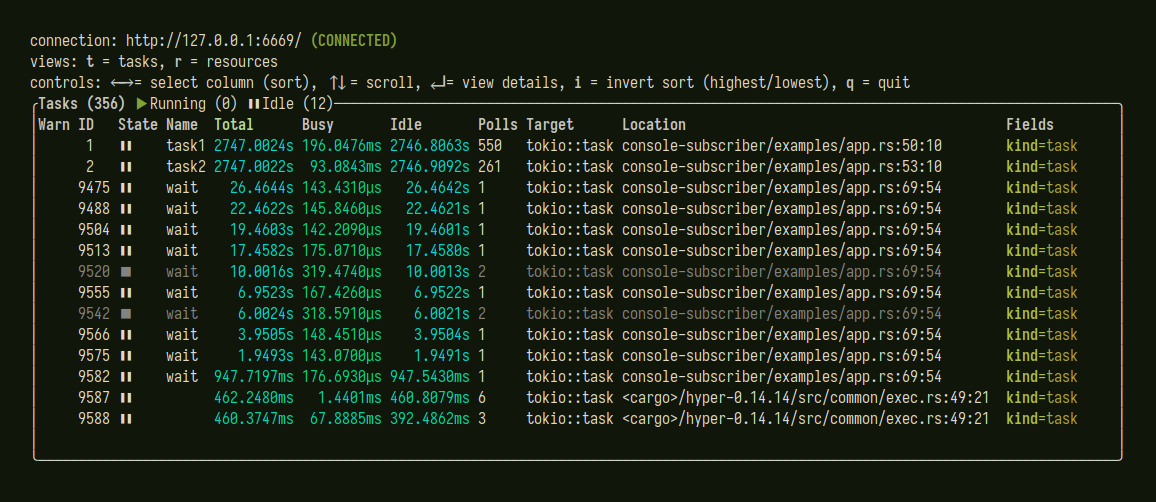 ...but if the terminal is too narrow, it wraps: 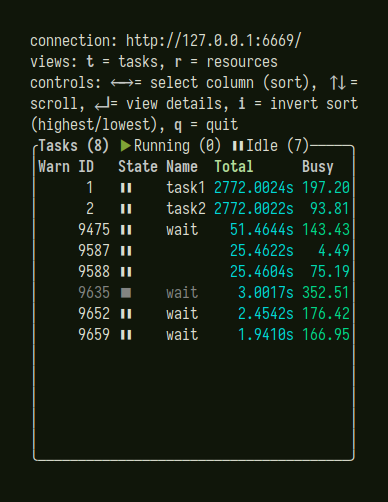 It might be nice to change this code to only wrap on commas, so each `<keys> = <description>` isn't broken...but that seems like a lot more work than just wrapping on whitespace, and the current thing is better than it was before!
- Loading branch information
Showing
4 changed files
with
69 additions
and
33 deletions.
There are no files selected for viewing
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters